CSCI 114 Programming Fundamentals II | Lab 3 Solitaire | Solution
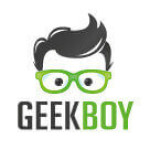
- ExpertT
- Rating : 109
- Grade : A+
- Questions : 1
- Solutions : 1026
- Blog : 0
- Earned : $53187.54
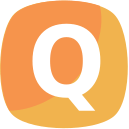
CSCI 114 Programming Fundamentals II
Purpose
The purpose of this lab is for you to work with array lists.
Problem specification
You will model a particular type of solitaire. The game starts with 45 cards. (They need
not be playing cards. Unmarked index cards work just as well.) Randomly divide them
into some number of piles of random size. For example, you might start with piles of
size 20, 5, 1, 9 and 10. In each round, you take one card from each pile, forming a new
pile with these cards. For example, the sample starting configuration would be
transformed into piles of size 19, 4, 8, 9 and 5. The solitaire is over when the piles have
size 1, 2, 3, 4, 5, 6, 7, 8, and 9, in some order. (It can be shown that you always end up
with such a configuration.)
You will write a Solitaire class to implement this behavior.
The SolitaireTester class
You are given a SolitaireTester class that uses Solitaire.
Source code is shown below, and can be downloaded from Blackboard, Course
Documents, Week 7 folder, Example programs. SolitaireTester code may not be
changed in any way.
public class SolitaireTester
{
public static void main(String[] args)
{
Solitaire s = new Solitaire();
System.out.println("Start: " + s.toString());
int rounds = 0;
while (!s.over()) {
s.round();
++rounds;
System.out.println(rounds + ": " + s.toString());
}
System.out.println("Rounds: " + rounds);
}
}
SolitaireTester produces a random starting configuration and prints it. Then keeps
applying the solitaire step and prints the result. Stops when the solitaire final
configuration is reached and prints the number of rounds.
A run of the program will look something like (many lines omitted for clarity):
Start: [11, 12, 22]
1: [10, 11, 21, 3]
2: [9, 10, 20, 2, 4]
3: [8, 9, 19, 1, 3, 5]
4: [7, 8, 18, 2, 4, 6]
5: [6, 7, 17, 1, 3, 5, 6]
6: [5, 6, 16, 2, 4, 5, 7]
7: [4, 5, 15, 1, 3, 4, 6, 7]
8: [3, 4, 14, 2, 3, 5, 6, 8]
9: [2, 3, 13, 1, 2, 4, 5, 7, 8]
10: [1, 2, 12, 1, 3, 4, 6, 7, 9]
. . .
55: [1, 2, 4, 4, 4, 6, 7, 8, 9]
56: [1, 3, 3, 3, 5, 6, 7, 8, 9]
57: [2, 2, 2, 4, 5, 6, 7, 8, 9]
58: [1, 1, 1, 3, 4, 5, 6, 7, 8, 9]
59: [2, 3, 4, 5, 6, 7, 8, 10]
60: [1, 2, 3, 4, 5, 6, 7, 9, 8]
Rounds: 60
The Solitaire class
Here are an outline and some hints for Solitaire:
Constants
as required
Exactly and only the following instance variable is required:
private ArrayList<Integer> piles;
constructor()
Initialize piles to a random number of piles of random size, but exactly 45 cards total.
Use Math.random() as you do this. random() returns a double value greater than or
equal to 0.0 and less than 1.0. As an example, to set num to a random integer value
between 1 and 10 inclusive is:
num = (int) (Math.random() * 10) + 1;
toString()
Return a string representation of a Solitaire object. To do this, simply:
return piles.toString();
over()
Return true if the solitaire is over, false otherwise.
The solitaire is over when the piles have size 1, 2, 3, 4, 5, 6, 7, 8, and 9, in some order.
(It can be shown that you always end up with such a configuration.)
round()
In each round, you take one card from each pile, forming a new pile with these cards.
Be very careful here when you remove a pile that reaches a size of 0 cards. Realize that
when you remove() an element from an ArrayList elements move down, changing their
indexes.
When you have run and tested your program and are certain it is correct, save the output
as an output.txt file. Obviously your start state will be random.
Required
Your program must look like the work of a professional computer programmer. You will
lose points if you do not meet ALL of the following requirements:
clear, consistent layout and indentation
use Javadoc documentation comments as you comment every class, every method and
anything that is not simple and clear
clear, meaningful variable and method names
design simple, clear algorithms
a method must do only a single task, and must be simple and short
use constants to avoid hard-coded magic numbers
Lab 3 submission
deadline for this lab is 3 weeks, by end of Sunday 3/15
Lab 3 Solitaire zip your BlueJ project plus output.txt output file and email to me at you will lose points if you do not include a file named output.txt containing the output of your program your email Subject line must say ‘CSCI 114 Lab 3’ followed by your full name, so that it filters to the correct email folder for grading you will lose points if you format your email Subject incorrectly e.g. my email Subject would be: this is a graded lab, so a reminder that you may not copy code from other people reminder that late labs will be penalized 2 points per week or part of week late
[Solved] CSCI 114 Programming Fundamentals II | Lab 3 Solitaire | Solution
- This Solution has been Purchased 1 time
- Submitted On 17 Mar, 2015 12:55:31
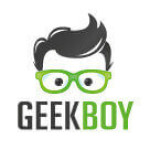
- ExpertT
- Rating : 109
- Grade : A+
- Questions : 1
- Solutions : 1026
- Blog : 0
- Earned : $53187.54
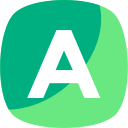
This Tutorial is rated A+ p...
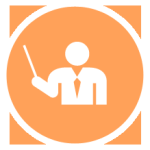
CSCI 114 Programming Fundamentals II | Lab 4 Wallet | Solution
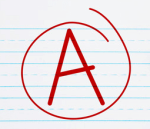
CSCI 114 Programming Fundamentals II Lab 5 Inheritance complete solutions correct answers key
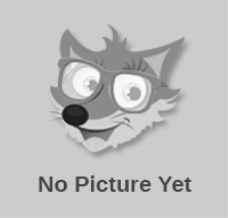
CSCI 114 Programming Fundamentals II | Lab 5 Inheritance | Solution
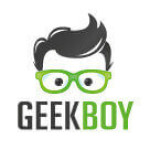
CSCI 114 Programming Fundamentals II | Lab 3 Solitaire | Solution
This Tutorial is rated A+ previously,if you have any questions regarding this tutorial then you can contact me.
...The benefits of buying study notes from CourseMerits
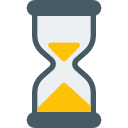
Assurance Of Timely Delivery
We value your patience, and to ensure you always receive your homework help within the promised time, our dedicated team of tutors begins their work as soon as the request arrives.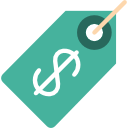
Best Price In The Market
All the services that are available on our page cost only a nominal amount of money. In fact, the prices are lower than the industry standards. You can always expect value for money from us.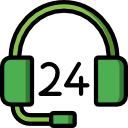