CSCI 114 Programming Fundamentals II Lab 5 Inheritance complete solutions correct answers key
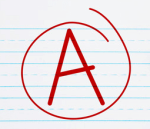
- Vpqnrqhwk
- Rating : 40
- Grade : A+
- Questions : 2
- Solutions : 1079
- Blog : 0
- Earned : $19352.58
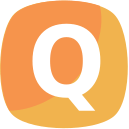
CSCI 114 Programming Fundamentals II Lab 5 Inheritance complete solutions correct answers key
Purpose
Purpose is to practice using regular inheritance.
The Checking superclass
First design, code and test a class called Checking. This will be your superclass.
Use exactly and only these instance variables:
private String accountID -an identifying account number e.g. “123”
private int balance -balance of the account in pennies, to avoid floating
point round-off errors e.g. 100 means $1.00
private int numDeposits -number of deposits made this month
private int numWithdrawals -number of withdrawals made this month
private double annualRate -annual interest rate e.g. 0.15 means 15% per
annum
private int serviceCharge -maintain the total monthly service charges incurred
here, in pennies
Checking will have the following methods. Be careful to implement the steps of each
method in exactly the order given below:
constructor
-has parameters for the account ID, starting balance (in dollars) and annual interest rate
-must initialize all instance variables
-use the following to convert the dollar starting balance parameter bal to cents, avoiding
round-off error:
balance = (int) Math.round(bal * 100.0);
appropriate get and set methods
-you have to decide during design what get and set methods are required
deposit()
-has a parameter for the deposit amount in dollars
-make the necessary conversion then add the amount to the balance
-increment the number of deposits
-add a deposit fee of 10 cents (in pennies) to the monthly service charge
© Anthony W. Smith, 2016
CSCI 114 Programming Fundamentals II
Lab 5 Inheritance
2
-note that the deposit fee is NOT deducted from balance at the time of the deposit
transaction
-(use static final constants for all the fee amounts)
withdraw()
-has a parameter for the withdrawal amount in dollars
-make the necessary conversion then subtract the amount from the balance
-increment the number of withdrawals
-add a withdrawal fee (in pennies) to the monthly service charge:
-the first 2 withdrawals cost 25 cents each
-withdrawals after the first 2 cost 75 cents each
depositInterest()
-update the balance by calculating the monthly interest earned on the account, then add
this interest to the balance. In pseudocode:
monthly interest rate = annual interest rate / 12.0
monthly interest = balance * monthly interest rate
balance = balance + monthly interest
-(use Math.round() where necessary to prevent rounding errors)
printMonthEnd()
-run at the end of the month to first add interest, then deduct monthly service charges,
than summarize the account. In pseudocode (implement these actions in exactly this
order):
call the depositInterest() method
add a monthly fee of $1.00 to the monthly service charge
decrease the balance by the total monthly service charge
output the account ID, resulting balance, number of deposits and withdrawals and the
monthly service charge (use printf() to output monetary amounts in dollars, with 2
decimal places)
set the number of deposits and withdrawals and monthly service charge to zero
-an example of the printMonthEnd() output format:
Account ID 123
Balance is: $751.88
1 deposits, 3 withdrawals
Monthly service charge was: $2.35
© Anthony W. Smith, 2016
CSCI 114 Programming Fundamentals II
Lab 5 Inheritance
3
toString()
-return a String that represents the Checking object, must be in standardized formatting
e.g.
Checking[accountID=123, balance=74800, numDeposits=1, numWithdrawals=3,
annualRate=0.1, serviceCharge=135]
The MoneyMarket subclass
Next design, code and test a MoneyMarket subclass that inherits from Checking.
MoneyMarket instance variable:
active a boolean variable that maintains the status of the account. Status is
defined as active if the balance is greater than a minimum balance of
$500.00, otherwise is not active. Account status must be updated
every time the account balance is changed. Withdrawals are not
allowed if the account is not active, until deposits make the account
active again
MoneyMarket will have the following methods Be careful to implement the steps of
each method in exactly the order given below:
constructor
-has parameters for account ID, the starting balance (in dollars) and annual interest rate
-use the superclass constructor to set these
-then set whether the account is active or not active
withdraw()
-output a message in the following format if the account is not active, and done:
MoneyMarket 456: Withdrawal not allowed
-otherwise call Checking withdraw() (use super to do this), then must update the status
of the account
deposit()
-call Checking deposit() (use super to do this), then must update the status of the
account
printMonthEnd()
-run at the end of the month to add interest, deduct monthly service charges and
summarize the account. In pseudocode (implement these actions in exactly this order):
© Anthony W. Smith, 2016
CSCI 114 Programming Fundamentals II
Lab 5 Inheritance
4
add a minimum balance fee of $5.00 to the monthly service fee if the account is not
active
call Checking monthEnd() (use super to do this)
update status of the account
output the status of the account e.g.
"Money market account is: active" or
"Money market account is: inactive"
-an example of the printMonthEnd() output format for the MoneyMarket class:
Account ID 456
Balance is: $296.15
1 deposits, 1 withdrawals
Monthly service charge was: $6.35
Money market account is: inactive
toString()
-return a String that represents the MoneyMarket object, must be in standardized
formatting e.g.
MoneyMarket[accountID=456, balance=30000, numDeposits=1,
numWithdrawals=1, annualRate=0.1, serviceCharge=35][active=false]
The Tester class
The Tester class tests your new Checking and MoneyMarket classes. Source code for
Tester is given below, and can be downloaded from Blackboard, Course Documents,
Week 12, Example programs.
import java.util.ArrayList;
/**
* Driver for Lab 5 Inheritance
*
* @author Anthony W. Smith
* @version 5/31/2028
*/
public class Tester
{
public static void main(String arg[])
{
// create some accounts
// $100.00, 10.0% annual interest
Checking check = new Checking("123", 100.0, 0.1);
// $1000.00, 10.0% annual interest
MoneyMarket money = new MoneyMarket("456", 1000.0, 0.1);
© Anthony W. Smith, 2016
CSCI 114 Programming Fundamentals II
Lab 5 Inheritance
5
ArrayList accounts = new ArrayList<>();
accounts.add(check);
accounts.add(money);
// do some transactions
System.out.println("Transactions");
// on Checking object
check.deposit(750.0);
check.withdraw(12.0);
check.withdraw(34.0);
check.withdraw(56.0);
// on MoneyMarket object
money.withdraw(750.0);
money.withdraw(100.0);
money.deposit(50.0);
// print accounts
System.out.println("\nPrint accounts");
for (Checking c : accounts)
System.out.println(c.toString());
// print month end report
System.out.println("\nMonth end report");
for (Checking c : accounts) {
c.printMonthEnd();
System.out.println();
}
}
}
Hints
first, take a calculator and work carefully through Tester line by line, writing down
on a piece of paper the values of the instance variables that should be produced...
this makes sure you understand what every method does, and gives you the expected
output you need to test your program
now design your new Checking and MoneyMarket classes on a piece of paper
use inheritance – a MoneyMarket account is-a Checking account, with an account
status added
design algorithms, think about parameters and return values
then use BlueJ as usual to code and test each method in turn. Comment out in Tester
the methods you have not yet implemented
© Anthony W. Smith, 2016
CSCI 114 Programming Fundamentals II
Lab 5 Inheritance
6
Required
Tester in your final submission must not be changed in any way
every method must have a clear, meaningful Javadoc comment
each toString() is required to use standardized formatting
automatically and routinely use all the other components of simplicity and clarity, as
listed in Blackboard, Course Information, “How labs are graded”
when you have thoroughly tested your program and are certain it is correct, save your
output into the output.txt file
Lab 5 submission
[Solved] CSCI 114 Programming Fundamentals II Lab 5 Inheritance complete solutions correct answers key
- This Solution has been Purchased 1 time
- Submitted On 01 May, 2016 07:40:21
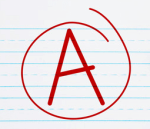
- Vpqnrqhwk
- Rating : 40
- Grade : A+
- Questions : 2
- Solutions : 1079
- Blog : 0
- Earned : $19352.58
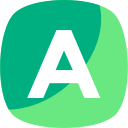
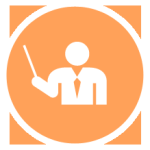
CSCI 114 Programming Fundamentals II | Lab 4 Wallet | Solution
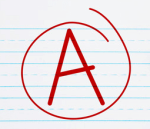
CSCI 114 Programming Fundamentals II Lab 5 Inheritance complete solutions correct answers key
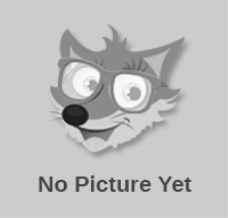
CSCI 114 Programming Fundamentals II | Lab 5 Inheritance | Solution
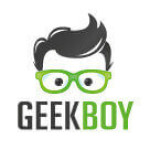
CSCI 114 Programming Fundamentals II | Lab 3 Solitaire | Solution
This Tutorial is rated A+ previously,if you have any questions regarding this tutorial then you can contact me.
...The benefits of buying study notes from CourseMerits
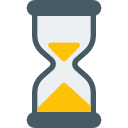
Assurance Of Timely Delivery
We value your patience, and to ensure you always receive your homework help within the promised time, our dedicated team of tutors begins their work as soon as the request arrives.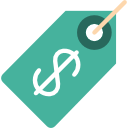
Best Price In The Market
All the services that are available on our page cost only a nominal amount of money. In fact, the prices are lower than the industry standards. You can always expect value for money from us.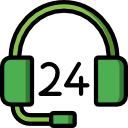