Project Solution C++
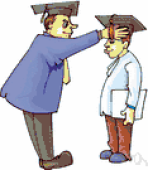
- Academia
- Rating : 60
- Grade : A+
- Questions : 0
- Solutions : 4595
- Blog : 1
- Earned : $25563.50
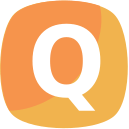
Project Solution
In this project, you will develop algorithms that find road routes through the bridges to travel between islands.
The input is a text file containing data about the given map. Each file begins with the number of rows and columns in the map considered as maximum latitudes and maximum longitudes respectively on the map. The character "X" in the file represents the water that means if a cell contains "X" then the traveler is not allowed to occupy that cell as this car is not drivable on water. The character "0" in the file represents the road connected island. That means if a cell contains "0" then the traveler is allowed to occupy that cell as this car can drive on roads.
The traveler starts at the island located at latitude = 0 and longitude = 0 (i.e., (0,0)) in the upper left corner, and the goal is to drive to the island located at (MaxLattitude-1, MaxLongitudes-1) in the lower right corner. A legal move from an island is to move left, right, up, or down to an immediately adjacent cell that has road connectivity which means a cell that contains "0". Moving off any edge of the map is not allowed.
Input: The map files
Output: Print paths as explicitly specified for all the functions in Part A, Part B, and extra credit on the console.
You should have single main function that calls all the required functions for Part A, Part B, and extra credit for all the 3 given input map files one by one.
Coded graph.h file for your reference and provided it for you to refer to and download on canvas. You do not have to use this file mandatory, but if you are struggling to even start the project then this should definitely make your life much easier.
Part A
Consider the following class map,
classmap{public:map(ifstream&fin);voidprint(int,int,int,int);boolisLegal(inti,intj);voidsetMap(inti,intj,intn);intgetMap(inti,intj)const;intgetReverseMapI(intn)const;intgetReverseMapJ(intn)const;voidmapToGraph(graph&g);boolfindPathRecursive(graph&g,stack&moves);boolfindPathNonRecursive1(graph&g,stack&moves);bool findPathNonRecursive2(graph &g, queue &moves);boolfindShortestPath1(graph&g,stack&bestMoves);boolfindShortestPath2(graph&,vector&bestMoves);voidmap::printPath(stack&s);intnumRows(){returnrows;};intnumCols(){returncols;};private:introws;// number of latitudes/rows in the mapintcols;// number of longitudes/columns in the mapmatrixvalue;matrixmapping;// Mapping from latitude and longitude co-ordinates (i,j) values to node index valuesvectorreverseMapI;// Mapping from node index values to map latitude i valuevectorreverseMapJ;// Mapping from node index values to map longitude j value};1. Using the above class map, write functionvoid map::mapToGraph(graph &g){...} to create a graph g that represents the legal moves in the map m. Each vertex should represent a cell, and each edge should represent a legal move between adjacent cells.2. Write a recursive functionfindPathRecursive(graph&g,stack&moves)that looks for a path from the start island to the destination island. If a path from the start to the destination exists, your function shouldcall the map::printPath() function that shouldprint a sequence of correct moves (Go left, Go right, Go down, Go up, etc.). If no path from the start to the destination exists, the program should print, "No path exists".If a solution exists the solver should also simulate the solution to each map by calling the map::print() function.The map::print() function prints out a map visualization, with the goal and current position of the car in the map at each move, marked to show the progress.Hint: consider recursive-DFS.3. Write a functionfindPathNonRecursive1(graph&g,stack&moves)that does the same thing as in 2, but by using stack and without using recursion. If a path from the start to the destination exists, your function shouldcall the map::printPath() function that shouldprint a sequence of correct moves (Go left, Go right, Go down, Go up, etc.). If no path from the start to the destination exists, the program should print, "No path exists".If a solution exists the solver should also simulate the solution to each map by calling the map::print() function.The map::print() function prints out a map visualization, with the goal and current position of the car in the map at each move, marked to show the progress.Hint: consider stack-based DFS.4. Write a function findPathNonRecursive2(graph &g, queue &moves) that does the same thing as in 2, but by using queue and without using recursion. If a path from the start to the destination exists, your function shouldcall the map::printPath() function that shouldprint a sequence of correct moves (Go left, Go right, Go down, Go up, etc.). If no path from the start to the destination exists, the program should print, "No path exists".If a solution exists the solver should also simulate the solution to each map by calling the map::print() function.The map::print() function prints out a map visualization, with the goal and current position of the car in the map at each move, marked to show the progress.Hint: consider queue-based BFS.
The code you submit should apply all three findPath functions to each map, one after the other.
The map input files named map1.txt, map2.txt, and map3.txt can be downloaded from the canvas. Example of a map input file:
7
10
Start - 0XXXXXXXXX
00000000XX
0X0X0X0XXX
0X0X0X0000
XX0XXX0XXX
X0000000XX
XXXXXXX000Z - Destination
Part B
The shortest path on a map is a path from the start to the destination with the smallest number of intermediate islands, that is the path with the least number of intermediate "0".
1. Write two functionsfindShortestPath1(graph&g,stack&bestMoves)andfindShortestPath2(graph&g,stack&bestMoves)that each finds the shortest path on a map if a path from the start to the destination exists. The first algorithm should use Dijkstra algorithm and the second algorithm should use Bellman-Ford algorithm to find the shortest paths. In each case, if a solution exists the solver shouldcall the map::printPath() function that shouldprint a sequence of correct moves (Go left, Go right, Go down, Go up, etc.). If no path from the start to the destination exists, the program should print, "No path exists".If a solution exists the solver should also simulate the solution to each map by calling the map::print() function.The map::print() function prints out a map visualization, with the goal and current position of the car in the map at each move, marked to show the progress.Each function should return true if any paths are found, and false otherwise.
Note: The use of the additional functions of class map is optional but highly recommended.
Extra Credit
1. Optimize Bellman-Ford algorithm to reduce the number of iterations and compare the runtime of the unmodified and modified algorithm for three given input maps. Your optimized Bellman-Ford algorithm shouldcall map::print() function. It should also print the runtime of the unoptimized and optimized versions.
Thus, You should submit a single zip folder within which you need to have 4 files including, 1) a single ".cpp" code file that includes all the above-required functions called from a single main function, 2) a single ".h" header file, 3) README file with the compile and execution instructions, and 4) your pre-compiled ".exe" executable file. On top of your .cpp code file, .h header file, and README file, you need to mention your name as comments. You may have an additional PDF file if you want to narrate your additional observations about running the functions of this project, e.g., drawing the graph to compare the runtime and explain the results. All submitted code files must be named as ".cpp". For example, it will be "jankibhimani.cpp", "jankibhimani_graph.h", and "jankibhimani.exe". The zip folder containing all the files should be named as "_code.zip". For example, it will be "jankibhimani_code.zip". Please follow the “Guidelines for Software Engineering Techniques.pdf” and “Assignments and Project Style and Documentation Guidelines.pdf” made available to you in your Student Resources module for other document editing and code format guidelines.
Some header files you may need are following, that can be found in you header files zip folder available to you to download on Canvas.
#include#include#include"d_except.h"#include#include#include"d_matrix.h"#include#include
[Solved] Project Solution C++
- This Solution has been Purchased 1 time
- Submitted On 18 Mar, 2022 04:55:44
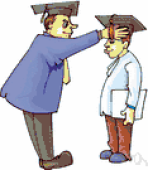
- Academia
- Rating : 60
- Grade : A+
- Questions : 0
- Solutions : 4595
- Blog : 1
- Earned : $25563.50
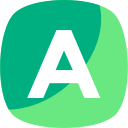
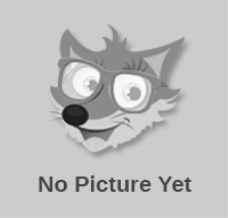
BUS 472L Unit 4 Discussion: Project Scheduling
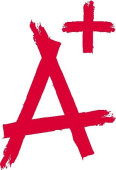
MASTERS___CJ510_FINAL_PROJECT_SUBMISSION.docx L.A. Riots Final Project Submission NAME
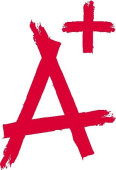
Final_Project_Submission_Comprehensive_Case_Study_Analysis.docx 7-1 Final Project Submi
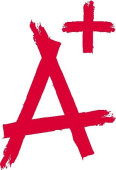
Final Audit Project.docx ACC 645 Final Audit Project Southern New Hampshire University A
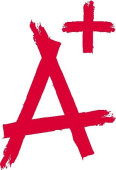
ACC 325 Accounting for Nonprofit Organizations Final Project Submission ACC 325: Accoun
The benefits of buying study notes from CourseMerits
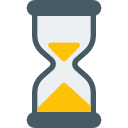
Assurance Of Timely Delivery
We value your patience, and to ensure you always receive your homework help within the promised time, our dedicated team of tutors begins their work as soon as the request arrives.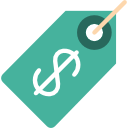
Best Price In The Market
All the services that are available on our page cost only a nominal amount of money. In fact, the prices are lower than the industry standards. You can always expect value for money from us.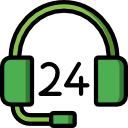