TEST BANK FOR Computer Systems A programmer’s perspective. 3rd Ed By Randal E. Bryant, David R.
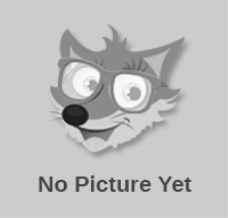
- A-Grades
- Rating : 0
- Grade : No Rating
- Questions : 0
- Solutions : 275
- Blog : 0
- Earned : $35.00
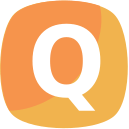
Computer Systems: A Programmer’s Perspective, Second Edition
Instructor’s Solution Manual, Version B 1
Randal E. Bryant
David R. O’Hallaron
February 4, 2010
1Copyright c 2010, R. E. Bryant, D. R. O’Hallaron. All rights reserved.
Chapter 1
Solutions to Homework Problems
This document contains solutions for the international version of the Second Edition of the book (we call
this “Version B”). A separate document contains solutions for the North American version (Version A).
The text uses two different kinds of exercises:
• Practice Problems. These are problems that are incorporated directly into the text, with explanatory
solutions at the end of each chapter. Our intention is that students will work on these problems as they
read the book. Each one highlights some particular concept.
• Homework Problems. These are found at the end of each chapter. They vary in complexity from
simple drills to multi-week labs and are designed for instructors to give as assignments or to use as
recitation examples.
This document gives the solutions to the homework problems.
1.1 Chapter 1: A Tour of Computer Systems
There are no homework problems in this chapter.
1.2 Chapter 2: Representing and Manipulating Information
Problem 2.57 Solution:
This exercise should be a straightforward variation on the existing code.
code/data/show-ans.c
1 void show_short(short x) {
2 show_bytes((byte_pointer) &x, sizeof(short));
3 }
4
3
4 CHAPTER 1. SOLUTIONS TO HOMEWORK PROBLEMS
5 void show_long(long x) {
6 show_bytes((byte_pointer) &x, sizeof(long));
7 }
8
9 void show_double(double x) {
10 show_bytes((byte_pointer) &x, sizeof(double));
11 }
code/data/show-ans.c
Problem 2.58 Solution:
There are many ways to solve this problem. The basic idea is to create some multibyte datum with different
values for the most and least-significant bytes. We then read byte 0 and determine which byte it is.
The following solution creates an int with value 1. We then access its first byte and convert it to an int.
This byte will equal 0 on a big-endian machine and 1 on a little-endian machine.
code/data/show-ans.c
1 int is_big_endian(void) {
2 /* MSB = 0, LSB = 1 */
3 int x = 1;
4 /* MSB (0) when big-endian, LSB (1) when little-endian */
5 char byte = *(char *) &x;
6 return !byte;
7 }
code/data/show-ans.c
Problem 2.59 Solution:
This is a simple exercise in masking and bit manipulation. It is important to mention that ˜0xFF is a way
to generate a mask that selects all but the least significant byte that works for any word size.
(x & ˜0xFF) | (y & 0xFF)
Problem 2.60 Solution:
Byte extraction and insertion code is useful in many contexts. Being able to write this sort of code is an
important skill to foster.
code/data/rbyte-ans.c
1 unsigned put_byte (unsigned x, unsigned char b, int i) {
2 int itimes8 = i << 3;
3 unsigned mask = 0xFF << itimes8;
4
5 return (x & ˜mask) | (b << itimes8);
6 }
code/data/rbyte-ans.c
1.2. CHAPTER 2: REPRESENTING AND MANIPULATING INFORMATION 5
Problem 2.61 Solution:
These exercises require thinking about the logical operation ! in a nontraditional way. Normally we think
of it as logical negation. More generally, it detects whether there is any nonzero bit in a word. In addition,
it gives practice with masking.
A. !!x
B. !!˜x
C. !!(x & (0xFF << ((sizeof(int)-1)<<3)))
D. !!(˜x & 0xFF)
Problem 2.62 Solution:
There are many solutions to this problem, but it is a little bit tricky to write one that works for any word
size. Here is our solution:
code/data/shift-ans.c
1 int int_shifts_are_logical() {
2 int x = -1; /* All 1’s */
3 return (x >> 1) > 0;
4 }
code/data/shift-ans.c
The above code performs a right shift of a word in which all bits are set to 1. If the shift is logical, the
resulting word will become positive.
Problem 2.63 Solution:
These problems are fairly tricky. They require generating masks based on the shift amounts. Shift value 0
must be handled as a special case, since otherwise we would be generating the mask by performing a left
shift by 32.
code/data/rshift-ans.c
1 int sra(int x, int k)
2 {
3 /* Perform shift logically */
4 int xsrl = (unsigned) x >> k;
5 /* Make mask of high order k bits */
6 unsigned mask = k ? ˜((1 << (8*sizeof(int)-k)) - 1) : 0;
7
8 return (x < 0) ? mask | xsrl : xsrl;
9 }
code/data/rshift-ans.c
code/data/rshift-ans.c
6 CHAPTER 1. SOLUTIONS TO HOMEWORK PROBLEMS
1 unsigned srl(unsigned x, int k)
2 {
3 /* Perform shift arithmetically */
4 unsigned xsra = (int) x >> k;
5 /* Make mask of low order w-k bits */
6 unsigned mask = k ? ((1 << (8*sizeof(int)-k)) - 1) : ˜0;
7
8 return xsra & mask;
9 }
code/data/rshift-ans.c
Problem 2.64 Solution:
This problem is very simple, but it reinforces the idea of using different bit patterns as masks.
code/data/bits.c
1 /* Return 1 when any even bit of x equals 1; 0 otherwise. Assume w=32 */
2 int any_even_one(unsigned x) {
3 /* Use mask to select even bits */
4 return (x&0x55555555) != 0;
5 }
code/data/bits.c
Problem 2.65 Solution:
This is a classic “bit puzzle” problem, and the solution is actually useful. The trick is to use the bit-level
parallelism of the ˆ operation to combine multiple bits at a time. The solution, using just 11 operations, is
as follows:
code/data/bits.c
1 /* Return 1 when x contains an even number of 1s; 0 otherwise. Assume w=32 */
2 int even_ones(unsigned x) {
3 /* Use bit-wise ˆ to compute multiple bits in parallel */
4 /* Xor bits i and i+16 for 0 <= i < 16 */
5 unsigned p16 = (x >> 16) ˆ x;
6 /* Xor bits i and i+8 for 0 <= i < 8 */
7 unsigned p8 = (p16>> 8) ˆ p16;
8 /* Xor bits i and i+4 for 0 <= i < 4 */
9 unsigned p4 = (p8 >> 4) ˆ p8;
10 /* Xor bits i and i+2 for 0 <= i < 2 */
11 unsigned p2 = (p4 >> 2) ˆ p4;
12 /* Xor bits 0 and 1 */
13 unsigned p1 = (p2 >> 1) ˆ p2;
14 /* Least significant bit is 1 if odd number of ones */
15 return !(p1 & 1);
16 }
[Solved] TEST BANK FOR Computer Systems A programmer’s perspective. 3rd Ed By Randal E. Bryant, David R.
- This solution is not purchased yet.
- Submitted On 10 Feb, 2022 12:41:42
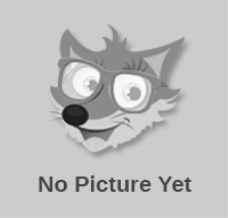
- A-Grades
- Rating : 0
- Grade : No Rating
- Questions : 0
- Solutions : 275
- Blog : 0
- Earned : $35.00
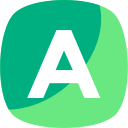
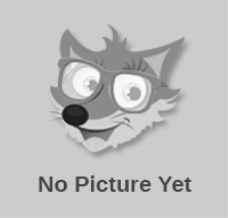
Health and Health Care Delivery in Canada 2nd Edition Test Bank
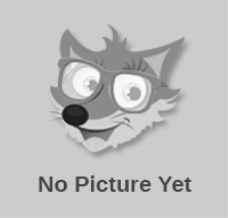
ATI Pharmacology Proctored Exam Test Bank
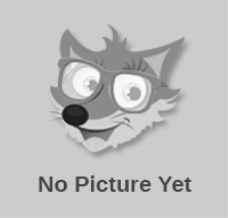
NCLEX RN EXAM {(TEST BANK) 4 VERSIONS} WITH 100% CORRECT QUESTIONS AND ANSWERS UPDATED 2024
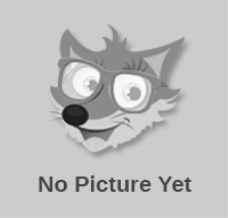
AQA AS PHYSICS 7407/1 Paper 1 Mark scheme (Test Bank) June 2024
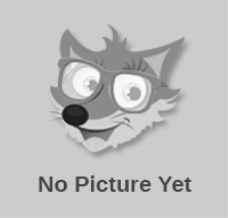
AQA AS PHYSICS 7407/1 Paper 1 Mark scheme (Test Bank) June 2024
The benefits of buying study notes from CourseMerits
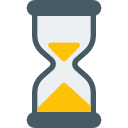
Assurance Of Timely Delivery
We value your patience, and to ensure you always receive your homework help within the promised time, our dedicated team of tutors begins their work as soon as the request arrives.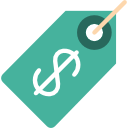
Best Price In The Market
All the services that are available on our page cost only a nominal amount of money. In fact, the prices are lower than the industry standards. You can always expect value for money from us.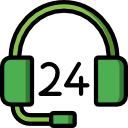