TEST BANK FOR Introduction to The Design and Analysis of Algorithms By Anany Levitin
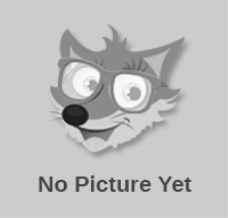
- A-Grades
- Rating : 0
- Grade : No Rating
- Questions : 0
- Solutions : 275
- Blog : 0
- Earned : $35.00
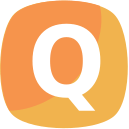
This file contains the exercises, hints, and solutions for Chapter 1 of the
book ”Introduction to the Design and Analysis of Algorithms,” 2nd edition, by
A. Levitin. The problems that might be challenging for at least some students
are marked by ; those that might be difficult for a majority of students are
marked by .
Exercises 1.1
1. Do some research on al-Khorezmi (also al-Khwarizmi), the man from
whose name the word “algorithm” is derived. In particular, you should
learn what the origins of the words “algorithm” and “algebra” have in
common.
2. Given that the official purpose of the U.S. patent system is the promotion
of the “useful arts,” do you think algorithms are patentable in this
country? Should they be?
3. a. Write down driving directions for going from your school to your home
with the precision required by an algorithm.
b. Write down a recipe for cooking your favorite dish with the precision
required by an algorithm.
4. Design an algorithm for computing
√
n for any positive integer n. Besides
assignment and comparison, your algorithm may only use the four
basic arithmetical operations.
5. a. Find gcd(31415, 14142) by applying Euclid’s algorithm.
b. Estimate how many times faster it will be to find gcd(31415, 14142)
by Euclid’s algorithm compared with the algorithm based on checking
consecutive integers from min{m, n} down to gcd(m, n).
6. Prove the equality gcd(m, n) = gcd(n,mmod n) for every pair of positive
integers m and n.
7. What does Euclid’s algorithm do for a pair of numbers in which the first
number is smaller than the second one? What is the largest number of
times this can happen during the algorithm’s execution on such an input?
8. a. What is the smallest number of divisions made by Euclid’s algorithm
among all inputs 1 ≤ m, n ≤ 10?
b. What is the largest number of divisions made by Euclid’s algorithm
among all inputs 1 ≤ m, n ≤ 10?
9. a. Euclid’s algorithm, as presented in Euclid’s treatise, uses subtractions
rather than integer divisions. Write a pseudocode for this version of
Euclid’s algorithm.
1
b. Euclid’s game (see [Bog]) starts with two unequal positive numbers
on the board. Two players move in turn. On each move, a player has
to write on the board a positive number equal to the difference of two
numbers already on the board; this number must be new, i.e., different
from all the numbers already on the board. The player who cannot move
loses the game. Should you choose to move first or second in this game?
10. The extended Euclid’s algorithm determines not only the greatest
common divisor d of two positive integers m and n but also integers (not
necessarily positive) x and y, such that mx + ny = d.
a. Look up a description of the extended Euclid’s algorithm (see, e.g.,
[KnuI], p. 13) and implement it in the language of your choice.
b. Modify your program for finding integer solutions to the Diophantine
equation ax + by = c with any set of integer coefficients a, b, and
c.
11. Locker doors There are n lockers in a hallway numbered sequentially
from 1 to n. Initially, all the locker doors are closed. You make n passes
by the lockers, each time starting with locker #1. On the ith pass, i =
1, 2, ..., n, you toggle the door of every ith locker: if the door is closed,
you open it, if it is open, you close it. For example, after the first pass
every door is open; on the second pass you only toggle the even-numbered
lockers (#2, #4, ...) so that after the second pass the even doors are
closed and the odd ones are opened; the third time through you close the
door of locker #3 (opened from the first pass), open the door of locker
#6 (closed from the second pass), and so on. After the last pass, which
locker doors are open and which are closed? How many of them are open?
2
Hints to Exercises 1.1
1. It is probably faster to do this by searching the Web, but your library
should be able to help too.
2. One can find arguments supporting either view. There is a well established
principle pertinent to the matter though: scientific facts or mathematical
expressions of them are not patentable. (Why do you think it is the case?)
But should this preclude granting patents for all algorithms?
3. You may assume that you are writing your algorithms for a human rather
than a machine. Still, make sure that your descriptions do not contain obvious
ambiguities. Knuth [KnuI], p.6 provides an interesting comparison
between cooking recipes and algorithms.
4. There is a quite straightforward algorithm for this problem based on the
definition of
√
n.
5. a. Just follow Euclid’s algorithm as described in the text.
b. Compare the number of divisions made by the two algorithms.
6. Prove that if d divides both m and n (i.e., m = sd and n = td for some
positive integers s and t), then it also divides both n and r = mmod n
and vice versa. Use the formula m = qn+r (0 ≤ r < n) and the fact that
if d divides two integers u and v, it also divides u+v and u−v. (Why?)
7. Perform one iteration of the algorithm for two arbitrarily chosen integers
m<n.
8. The answer to part (a) can be given immediately; the answer to part
(b) can be given by checking the algorithm’s performance on all pairs
1<m< n ≤ 10.
9. a. Use the equality
gcd(m, n) = gcd(m − n, n) for m ≥ n > 0.
b. The key is to figure out the total number of distinct integers that can be
written on the board, starting with an initial pair m, n where m > n ≥ 1.
You should exploit a connection of this question to the question of part
(a). Considering small examples, especially those with n = 1 and n = 2,
should help, too.
10. Of course, for some coefficients, the equation will have no solutions.
11. Tracing the algorithm by hand for, say, n = 10 and studying its outcome
should help answering both questions.
3
Solutions to Exercises 1.1
1. Al-Khwarizmi (9th century C.E.) was a great Arabic scholar, most famous
for his algebra textbook. In fact, the word “algebra” is derived
from the Arabic title of this book while the word “algorithm” is derived
from a translation of Al-Khwarizmi’s last name (see, e.g., [KnuI], pp. 1-2,
[Knu96], pp. 88-92, 114).
2. This legal issue has yet to be settled. The current legal state of affairs
distinguishes mathematical algorithms, which are not patentable, from
other algorithms, which may be patentable if implemented as computer
programs (e.g., [Cha00]).
3. n/a
4. A straightforward algorithm that does not rely on the availability of an
approximate value of
√
n can check the squares of consecutive positive
integers until the first square exceeding n is encountered. The answer will
be the number’s immediate predecessor. Note: A much faster algorithm
for solving this problem can be obtained by using Newton’s method (see
Sections 11.4 and 12.4).
5. a. gcd(31415, 14142) = gcd(14142, 3131) = gcd(3131, 1618) =
gcd(1618, 1513) = gcd(1513, 105) = gcd(1513, 105) = gcd(105, 43) =
gcd(43, 19) = gcd(19, 5) = gcd(5, 4) = gcd(4, 1) = gcd(1, 0) = 1.
b. To answer the question, we need to compare the number of divisions
the algorithms make on the input given. The number of divisions made
by Euclid’s algorithm is 11 (see part a). The number of divisions made
by the consecutive integer checking algorithm on each of its 14142 iterations
is either 1 and 2; hence the total number of multiplications is between
1·14142 and 2·14142. Therefore, Euclid’s algorithm will be between
1·14142/11 ≈ 1300 and 2·14142/11 ≈ 2600 times faster.
6. Let us first prove that if d divides two integers u and v, it also divides
both u+v and u−v. By definition of division, there exist integers s and
t such that u = sd and v = td. Therefore
u ± v = sd ± td = (s ± t)d,
i.e., d divides both u + v and u − v.
4
Also note that if d divides u, it also divides any integer multiple ku of
u. Indeed, since d divides u, u = sd. Hence
ku = k(sd) = (ks)d,
i.e., d divides ku.
Now we can prove the assertion in question. For any pair of positive
integers m and n, if d divides both m and n, it also divides both n and
r = mmod n = m−qn. Similarly, if d divides both n and r = mmod n =
m − qn, it also divides both m = r + qn and n. Thus, the two pairs
(m, n) and (n, r) have the same finite nonempty set of common divisors,
including the largest element in the set, i.e., gcd(m, n) = gcd(n, r).
7. For any input pair m, n such that 0 ≤ m < n, Euclid’s algorithm simply
swaps the numbers on the first iteration:
gcd(m, n) = gcd(n,m)
because mmod n = m if m < n. Such a swap can happen only once since
gcd(m, n) = gcd(n,mmod n) implies that the first number of the new pair
(n) will be greater than its second number (mmod n) after every iteration
of the algorithm.
8. a. For any input pair m ≥ n ≥ 1, in which m is a multiple of n, Euclid’s
algorithm makes exactly one division; it is the smallest number possible
for two positive numbers.
b. The answer is 5 divisions, which is made by Euclid’s algorithm in
computing gcd(5, 8). It is not too time consuming to get this answer by
examining the number of divisions made by the algorithm on all input
pairs 1 < m < n ≤ 10.
Note: A pertinent general result (see [KnuII], p. 360) is that for any
input pair m, n where 0 ≤ n < N, the number of divisions required by
Euclid’s algorithm to compute gcd(m, n) is at most logφ(3−φ)N) where
φ = (1+
√
5)/2.
9. a. Here is a nonrecursive version:
Algorithm Euclid2 (m, n)
//Computes gcd(m, n) by Euclid’s algorithm based on subtractions
//Input: Two nonnegative integers m and n not both equal to 0
//Output: The greatest common divisor of m and n
while n = 0 do
if m < n swap(m, n)
m ← m− n
return m
5
b. It is not too difficult to prove that the integers that can be written on
the board are the integers generated by the subtraction version of Euclid’s
algorithm and only them. Although the order in which they appear on
the board may vary, their total number always stays the same: It is equal
to m/gcd(m, n), where m is the maximum of the initial numbers, which
includes two integers of the initial pair. Hence, the total number of
possible moves is m/gcd(m, n)−2. Consequently, if m/gcd(m, n) is odd,
one should choose to go first; if it is even, one should choose to go second.
10. n/a
11. Since all the doors are initially closed, a door will be open after the last
pass if and only if it is toggled an odd number of times. Door i (1 ≤ i ≤ n)
is toggled on pass j (1 ≤ j ≤ n) if and only if j divides i. Hence, the total
number of times door i is toggled is equal to the number of its divisors.
Note that if j divides i, i.e. i = jk, then k divides i too. Hence all the
divisors of i can be paired (e.g., for i = 12, such pairs are 1 and 12, 2
and 6, 3 and 4) unless i is a perfect square (e.g., for i = 16, 4 does not
have another divisor to be matched with). This implies that i has an
odd number of divisors if and only if it is a perfect square, i.e., i = j2.
Hence doors that are in the positions that are perfect squares and only
such doors will be open after the last pass. The total number of such
positions not exceeding n is equal to
√
n: these numbers are the squares
of the positive integers between 1 and
√
n inclusively.
6
Exercises 1.2
1. Old World puzzle A peasant finds himself on a riverbank with a wolf,
a goat, and a head of cabbage. He needs to transport all three to the
other side of the river in his boat. However, the boat has room for only
the peasant himself and one other item (either the wolf, the goat, or the
cabbage). In his absence, the wolf would eat the goat, and the goat would
eat the cabbage. Solve this problem for the peasant or prove it has no
solution. (Note: The peasant is a vegetarian but does not like cabbage
and hence can eat neither the goat nor the cabbage to help him solve the
problem. And it goes without saying that the wolf is a protected species.)
2. New World puzzle There are four people who want to cross a bridge; they
all begin on the same side. You have 17 minutes to get them all across to
the other side. It is night, and they have one flashlight. A maximum of two
people can cross the bridge at one time. Any party that crosses, either one
or two people, must have the flashlight with them. The flashlight must be
walked back and forth; it cannot be thrown, for example. Person 1 takes
1 minute to cross the bridge, person 2 takes 2 minutes, person 3 takes 5
minutes, and person 4 takes 10 minutes. A pair must walk together at the
rate of the slower person’s pace. For example, if person 1 and person 4
walk across first, 10 minutes have elapsed when they get to the other side
of the bridge. If person 4 returns the flashlight, a total of 20 minutes have
passed and you have failed the mission. (Note: According to a rumor on
the Internet, interviewers at a well-known software company located near
Seattle have given this problem to interviewees.)
3. Which of the following formulas can be considered an algorithm for computing
the area of a triangle whose side lengths are given positive numbers
a, b, and c?
a. S = p(p − a)(p − b)(p − c), where p = (a + b + c)/2
b. S = 1
2 bc sin A, where A is the angle between sides b and c
c. S = 1
2aha, where ha is the height to base a
4. Write a pseudocode for an algorithm for finding real roots of equation
ax2 + bx + c = 0 for arbitrary real coefficients a, b, and c. (You may
assume the availability of the square root function sqrt(x).)
5. Describe the standard algorithm for finding the binary representation of
a positive decimal integer
a. in English.
b. in a pseudocode.
[Solved] TEST BANK FOR Introduction to The Design and Analysis of Algorithms By Anany Levitin
- This solution is not purchased yet.
- Submitted On 09 Feb, 2022 10:24:39
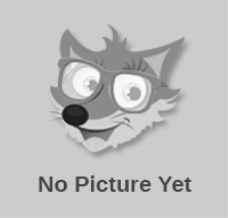
- A-Grades
- Rating : 0
- Grade : No Rating
- Questions : 0
- Solutions : 275
- Blog : 0
- Earned : $35.00
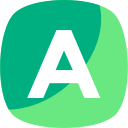
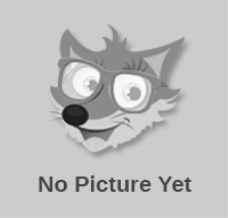
Health and Health Care Delivery in Canada 2nd Edition Test Bank
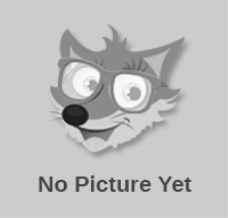
ATI Pharmacology Proctored Exam Test Bank
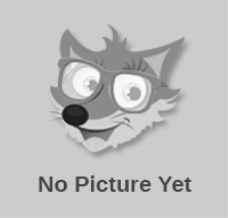
Medical Surgical Nursing 2nd Edition Hoffman Test Bank

COMPLETE HESI Exit Exam Test Bank, All Versions Covered 100%GRADED A+ WIT
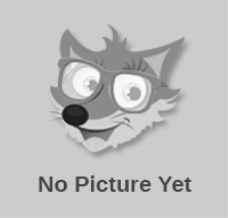
Med Surg ATI Proctored Exam Test Bank 2023 With NGN
The benefits of buying study notes from CourseMerits
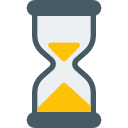
Assurance Of Timely Delivery
We value your patience, and to ensure you always receive your homework help within the promised time, our dedicated team of tutors begins their work as soon as the request arrives.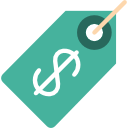
Best Price In The Market
All the services that are available on our page cost only a nominal amount of money. In fact, the prices are lower than the industry standards. You can always expect value for money from us.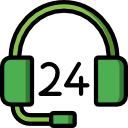