TEST BANK Annotated Solution Guide for Thinking in Java 4th Edition By Bruce Eckel and Ervin Varga
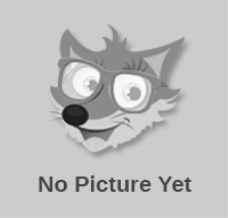
- A-Grades
- Rating : 0
- Grade : No Rating
- Questions : 0
- Solutions : 275
- Blog : 0
- Earned : $35.00
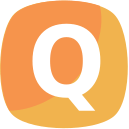
Operators
To satisfy IDEs like Eclipse, we have included package statements for chapters
before Access Control. If you have solved the problems in this chapter without
using package statements, your solutions are still correct.
Exercise 1
//: operators/E01_PrintStatements.java
/****************** Exercise 1 *****************
* Write a program that uses the "short" and
* normal form of print statement.
***********************************************/
package operators;
import java.util.Date;
import static net.mindview.util.Print.*;
public class E01_PrintStatements {
public static void main(String[] args) {
Date currDate = new Date();
System.out.println("Hello, it's: " + currDate);
print("Hello, it's: " + currDate);
}
} /* Output: (Sample)
Hello, it's: Wed Mar 30 17:39:26 CEST 2005
Hello, it's: Wed Mar 30 17:39:26 CEST 2005
*///:~
Exercise 2
//: operators/E02_Aliasing.java
/****************** Exercise 2 *****************
* Create a class containing a float and use it to
* demonstrate aliasing.
***********************************************/
package operators;
import static net.mindview.util.Print.*;
class Integral {
float f;
}
22 Thinking in Java, 4th Edition Annotated Solution Guide
public class E02_Aliasing {
public static void main(String[] args) {
Integral n1 = new Integral();
Integral n2 = new Integral();
n1.f = 9f;
n2.f = 47f;
print("1: n1.f: " + n1.f + ", n2.f: " + n2.f);
n1 = n2;
print("2: n1.f: " + n1.f + ", n2.f: " + n2.f);
n1.f = 27f;
print("3: n1.f: " + n1.f + ", n2.f: " + n2.f);
}
} /* Output:
1: n1.f: 9.0, n2.f: 47.0
2: n1.f: 47.0, n2.f: 47.0
3: n1.f: 27.0, n2.f: 27.0
*///:~
You can see the effect of aliasing after n2 is assigned to n1: they both point to
the same object.
Exercise 3
//: operators/E03_Aliasing2.java
/****************** Exercise 3 *****************
* Create a class containing a float and use it
* to demonstrate aliasing during method calls.
***********************************************/
package operators;
import static net.mindview.util.Print.*;
public class E03_Aliasing2 {
static void f(Integral y) { y.f = 1.0f; }
public static void main(String[] args) {
Integral x = new Integral();
x.f = 2.0f;
print("1: x.f: " + x.f);
f(x);
print("2: x.f: " + x.f);
}
} /* Output:
1: x.f: 2.0
2: x.f: 1.0
*///:~
Operators 23
This exercise emphasizes that you’re always passing references around, thus
you’re always aliasing. Even when you don’t actually see changes being made to
the code you’re writing or the method you’re calling, that code or method could
be calling other methods that modify the object.
Exercise 4
//: operators/E04_Velocity.java
// {Args: 30.5 3.2}
/****************** Exercise 4 *****************
* Write a program that calculates velocity
* using a constant distance and a constant time.
***********************************************/
package operators;
public class E04_Velocity {
public static void main(String[] args) {
if(args.length < 2) {
System.err.println(
"Usage: java E04_Velocity distance time");
System.exit(1);
}
float distance = Float.parseFloat(args[0]);
float time = Float.parseFloat(args[1]);
System.out.print("Velocity = ");
System.out.print(distance / time);
// Change the next line if you want to use a different
// unit for 'distance'
System.out.println(" m/s");
}
} /* Output:
Velocity = 9.53125 m/s
*///:~
Here we take the distance and time values from the command line. Arguments
come in as a String array; if you need a float instead, use the static
parseFloat( ) method of class Float. This can be difficult to locate using the
JDK HTML documentation; you must remember either “parse” or that it’s part
of class Float.
Note the difference between System.out.print( ) and
System.out.println( ); the latter terminates the current line by writing the
line separator string.
24 Thinking in Java, 4th Edition Annotated Solution Guide
Exercise 5
//: operators/E05_Dogs.java
/****************** Exercise 5 *****************
* Create a class called Dog with two Strings:
* name and says. In main(), create two dogs,
* "spot" who says, "Ruff!", and "scruffy" who
* says, "Wurf!". Then display their names and
* what they say.
***********************************************/
package operators;
class Dog {
String name;
String says;
}
public class E05_Dogs {
public static void main(String[] args) {
Dog dog1 = new Dog();
Dog dog2 = new Dog();
dog1.name = "spot"; dog1.says = "ruff!";
dog2.name = "scruffy"; dog2.says = "wurf!";
System.out.println(dog1.name + " says " + dog1.says);
System.out.println(dog2.name + " says " + dog2.says);
}
} /* Output:
spot says ruff!
scruffy says wurf!
*///:~
This walks you through the basics of objects, and demonstrates that each object
has its own distinct storage space.
Exercise 6
//: operators/E06_DogsComparison.java
/****************** Exercise 6 *****************
* Following Exercise 5 assign, a new Dog
* reference to spot's object. Test for comparison
* using == and equals() for all references.
***********************************************/
package operators;
import static net.mindview.util.Print.*;
Operators 25
public class E06_DogsComparison {
static void compare(Dog dog1, Dog dog2) {
print("== on top references: " + (dog1 == dog2));
print(
"equals() on top references: " + dog1.equals(dog2)
);
print("== on names: " + (dog1.name == dog2.name));
print(
"equals() on names: " + dog1.name.equals(dog2.name)
);
print("== on says: " + (dog1.says == dog2.says));
print(
"equals() on says: " + dog1.says.equals(dog2.says)
);
}
public static void main(String[] args) {
Dog dog1 = new Dog();
Dog dog2 = new Dog();
Dog dog3 = dog1; // "Aliased" reference
dog1.name = "spot"; dog1.says = "ruff!";
dog2.name = "scruffy"; dog2.says = "wurf!";
print("Comparing dog1 and dog2 objects...");
compare(dog1, dog2);
print("\nComparing dog1 and dog3 objects...");
compare(dog1, dog3);
print("\nComparing dog2 and dog3 objects...");
compare(dog2, dog3);
}
} /* Output:
Comparing dog1 and dog2 objects...
== on top references: false
equals() on top references: false
== on names: false
equals() on names: false
== on says: false
equals() on says: false
Comparing dog1 and dog3 objects...
== on top references: true
equals() on top references: true
== on names: true
equals() on names: true
== on says: true
equals() on says: true
Comparing dog2 and dog3 objects...
== on top references: false
26 Thinking in Java, 4th Edition Annotated Solution Guide
equals() on top references: false
== on names: false
equals() on names: false
== on says: false
equals() on says: false
*///:~
Guess whether the following line compiles:
print("== on top references: " + dog1 == dog2);
Why or why not? (Hint: Read the Precedence and String operator + and +=
sections in TIJ4.) Apply the same reasoning to the next case and explain why the
comparison always results in false:
print("== on says: " + dog1.name == dog2.name);
Exercise 7
//: operators/E07_CoinFlipping.java
/****************** Exercise 7 *****************
* Write a program that simulates coin-flipping.
***********************************************/
package operators;
import java.util.Random;
public class E07_CoinFlipping {
public static void main(String[] args) {
Random rand = new Random(47);
boolean flip = rand.nextBoolean();
System.out.print("OUTCOME: ");
System.out.println(flip ? "HEAD" : "TAIL");
}
} /* Output:
OUTCOME: HEAD
*///:~
This is partly an exercise in Standard Java Library usage. After familiarizing
yourself with the HTML documentation for the JDK (downloadable from
java.sun.com), select “R” at the JDK index to see various ways to generate
random numbers.
The program uses a ternary if-else operator to produce output. (See the Ternary
if-else Operator section in TIJ4 for more information.)
NOTE: You will normally create a Random object with no arguments to
produce different output for each execution of the program. Otherwise it can
Operators 27
hardly be called a simulator. In this exercise and throughout the book, we use
the seed value of 47 to make the output identical, thus verifiable, for each run.
Exercise 8
//: operators/E08_LongLiterals.java
/****************** Exercise 8 *****************
* Show that hex and octal notations work with long
* values. Use Long.toBinaryString() to display
* the results.
***********************************************/
package operators;
import static net.mindview.util.Print.*;
public class E08_LongLiterals {
public static void main(String[] args) {
long l1 = 0x2f; // Hexadecimal (lowercase)
print("l1: " + Long.toBinaryString(l1));
long l2 = 0X2F; // Hexadecimal (uppercase)
print("l2: " + Long.toBinaryString(l2));
long l3 = 0177; // Octal (leading zero)
print("l3: " + Long.toBinaryString(l3));
}
} /* Output:
l1: 101111
l2: 101111
l3: 1111111
*///:~
Note that Long.toBinaryString( ) does not print leading zeroes.
Exercise 9
//: operators/E09_MinMaxExponents.java
/****************** Exercise 9 *****************
* Display the largest and smallest numbers for
* both float and double exponential notation.
***********************************************/
package operators;
import static net.mindview.util.Print.*;
public class E09_MinMaxExponents {
public static void main(String[] args) {
print("Float MIN: " + Float.MIN_VALUE);
print("Float MAX: " + Float.MAX_VALUE);
28 Thinking in Java, 4th Edition Annotated Solution Guide
print("Double MIN: " + Double.MIN_VALUE);
print("Double MAX: " + Double.MAX_VALUE);
}
} /* Output:
Float MIN: 1.4E-45
Float MAX: 3.4028235E38
Double MIN: 4.9E-324
Double MAX: 1.7976931348623157E308
*///:~
Exercise 10
//: operators/E10_BitwiseOperators.java
/****************** Exercise 10 *****************
* Write a program with two constant values, one
* with alternating binary ones and zeroes, with
* a zero in the least-significant digit, and the
* second, also alternating, with a one in the
* least-significant digit. (Hint: It's easiest to
* use hexadecimal constants for this.) Combine
* these two values every way possible using the
* bitwise operators. Display the results using
* Integer.toBinaryString().
************************************************/
package operators;
import static net.mindview.util.Print.*;
public class E10_BitwiseOperators {
public static void main(String[] args) {
int i1 = 0xaaaaaaaa;
int i2 = 0x55555555;
print("i1 = " + Integer.toBinaryString(i1));
print("i2 = " + Integer.toBinaryString(i2));
print("~i1 = " + Integer.toBinaryString(~i1));
print("~i2 = " + Integer.toBinaryString(~i2));
print("i1 & i1 = " + Integer.toBinaryString(i1 & i1));
print("i1 | i1 = " + Integer.toBinaryString(i1 | i1));
print("i1 ^ i1 = " + Integer.toBinaryString(i1 ^ i1));
print("i1 & i2 = " + Integer.toBinaryString(i1 & i2));
print("i1 | i2 = " + Integer.toBinaryString(i1 | i2));
print("i1 ^ i2 = " + Integer.toBinaryString(i1 ^ i2));
}
} /* Output:
i1 = 10101010101010101010101010101010
i2 = 1010101010101010101010101010101
~i1 = 1010101010101010101010101010101
Operators 29
~i2 = 10101010101010101010101010101010
i1 & i1 = 10101010101010101010101010101010
i1 | i1 = 10101010101010101010101010101010
i1 ^ i1 = 0
i1 & i2 = 0
i1 | i2 = 11111111111111111111111111111111
i1 ^ i2 = 11111111111111111111111111111111
*///:~
Note that Integer.toBinaryString( ) does not print leading zeroes.
Exercise 11
//: operators/E11_SignedRightShift.java
/****************** Exercise 11 *****************
* Start with a number that has a binary one in
* the most significant position. (Hint: Use a
* hexadecimal constant.) Use the signed
* right-shift operator to right shift your
* number through all its binary positions.
* Display each result using Integer.toBinaryString().
************************************************/
package operators;
import static net.mindview.util.Print.*;
public class E11_SignedRightShift {
public static void main(String[] args) {
int i = 0x80000000;
print(Integer.toBinaryString(i));
i >>= 1; print(Integer.toBinaryString(i));
i >>= 1; print(Integer.toBinaryString(i));
i >>= 1; print(Integer.toBinaryString(i));
i >>= 1; print(Integer.toBinaryString(i));
i >>= 1; print(Integer.toBinaryString(i));
i >>= 1; print(Integer.toBinaryString(i));
i >>= 1; print(Integer.toBinaryString(i));
i >>= 1; print(Integer.toBinaryString(i));
i >>= 1; print(Integer.toBinaryString(i));
i >>= 1; print(Integer.toBinaryString(i));
i >>= 1; print(Integer.toBinaryString(i));
i >>= 1; print(Integer.toBinaryString(i));
i >>= 1; print(Integer.toBinaryString(i));
i >>= 1; print(Integer.toBinaryString(i));
i >>= 1; print(Integer.toBinaryString(i));
i >>= 1; print(Integer.toBinaryString(i));
i >>= 1; print(Integer.toBinaryString(i));
30 Thinking in Java, 4th Edition Annotated Solution Guide
i >>= 1; print(Integer.toBinaryString(i));
i >>= 1; print(Integer.toBinaryString(i));
i >>= 1; print(Integer.toBinaryString(i));
i >>= 1; print(Integer.toBinaryString(i));
i >>= 1; print(Integer.toBinaryString(i));
i >>= 1; print(Integer.toBinaryString(i));
i >>= 1; print(Integer.toBinaryString(i));
i >>= 1; print(Integer.toBinaryString(i));
i >>= 1; print(Integer.toBinaryString(i));
i >>= 1; print(Integer.toBinaryString(i));
i >>= 1; print(Integer.toBinaryString(i));
i >>= 1; print(Integer.toBinaryString(i));
i >>= 1; print(Integer.toBinaryString(i));
i >>= 1; print(Integer.toBinaryString(i));
}
} /* Output:
10000000000000000000000000000000
11000000000000000000000000000000
11100000000000000000000000000000
11110000000000000000000000000000
11111000000000000000000000000000
11111100000000000000000000000000
11111110000000000000000000000000
11111111000000000000000000000000
11111111100000000000000000000000
11111111110000000000000000000000
11111111111000000000000000000000
11111111111100000000000000000000
11111111111110000000000000000000
11111111111111000000000000000000
11111111111111100000000000000000
11111111111111110000000000000000
11111111111111111000000000000000
11111111111111111100000000000000
11111111111111111110000000000000
11111111111111111111000000000000
11111111111111111111100000000000
11111111111111111111110000000000
11111111111111111111111000000000
11111111111111111111111100000000
11111111111111111111111110000000
11111111111111111111111111000000
11111111111111111111111111100000
11111111111111111111111111110000
11111111111111111111111111111000
11111111111111111111111111111100
11111111111111111111111111111110
Operators 31
11111111111111111111111111111111
*///:~
This exercise required duplicating the line of code (the “brute force” approach).
In the next chapter you learn to use looping structures to greatly reduce the
amount of code you see above.
[Solved] TEST BANK Annotated Solution Guide for Thinking in Java 4th Edition By Bruce Eckel and Ervin Varga
- This solution is not purchased yet.
- Submitted On 06 Feb, 2022 10:52:26
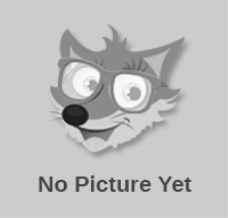
- A-Grades
- Rating : 0
- Grade : No Rating
- Questions : 0
- Solutions : 275
- Blog : 0
- Earned : $35.00
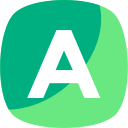
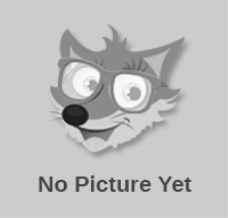
Health and Health Care Delivery in Canada 2nd Edition Test Bank
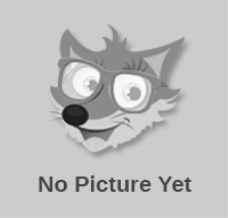
ATI Pharmacology Proctored Exam Test Bank

Physical Problems, Psychological Sources Test Bank

Physical Problems, Psychological Sources Test Bank

Test Bank For Pharmacotherapeutics for Advanced Practice Nurse Pharmacotherapy
The benefits of buying study notes from CourseMerits
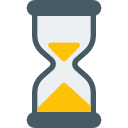
Assurance Of Timely Delivery
We value your patience, and to ensure you always receive your homework help within the promised time, our dedicated team of tutors begins their work as soon as the request arrives.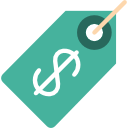
Best Price In The Market
All the services that are available on our page cost only a nominal amount of money. In fact, the prices are lower than the industry standards. You can always expect value for money from us.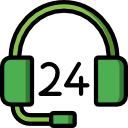