IT213 Unit 3 Assignment | Complete Solution
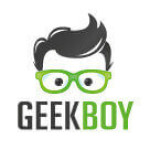
- ExpertT
- Rating : 109
- Grade : A+
- Questions : 1
- Solutions : 1026
- Blog : 0
- Earned : $53187.54
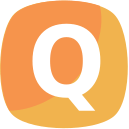
Unit 3 [IT213: Software Development Concepts]
Unit 3 Assignment Instructions
In this unit you will complete the coding exercises before starting the Coding Project. Review the
detailed instructions and rubrics before starting your Assignments.
Submission Instructions
You will submit the following:
.cs files for C#
.java files for Java
.php and/or .js files for Web Development
Additionally, in a Word document, paste a screenshot of the output for each exercise.
You will be submitting three code files, one for each exercise (.cs, .java, .php or .js) and one Word
document in a zipped folder.
Zip these four files into a zipped folder and submit the one zipped folder.
Naming Your Files and Zip Folder
The code files should be saved as: IT213_YourLastName_UnitX_ExerciseX_Language.
The word document should be saved as: IT213_YourLastName_UnitX_Screenshots
The zip folder should be saved as: IT213_YourLastName_UnitX_ZIP
Unit 3 Assignment: Coding Exercises
You must complete the following coding exercises before starting the two Coding Projects. By
completing these exercises you will be better prepared for the Assignment.
Note: If your language of choice is Web Development, you will need to complete the exercises in both
PHP and JavaScript.
3-1. Class Grade Average The following code is shown for Java, C#, and JavaScript. For the
language of your choice, type in the following code and run it."
Java
Unit 3 [IT213: Software Development Concepts]
// GradeBook class that solves class-average problem using
// counter-controlled repetition.
import java.util.Scanner; // program uses class Scanner
public class GradeBook
{
public static void main(String[] args){
determineClassAverage();
}
// determine class average based on 10 grades entered by user
public static void determineClassAverage()
{
// create Scanner to obtain input from command window
Scanner input = new Scanner( System.in );
int total; // sum of grades entered by user
int gradeCounter; // number of the grade to be entered next
int grade; // grade value entered by user
int average; // average of grades
// initialization phase
total = 0; // initialize total
gradeCounter = 1; // initialize loop counter
// processing phase uses counter-controlled repetition
Unit 3 [IT213: Software Development Concepts]
while ( gradeCounter <= 10 ) // loop 10 times
{
System.out.print( "Enter grade: " ); // prompt
grade = input.nextInt(); // input next grade
total = total + grade; // add grade to total
gradeCounter = gradeCounter + 1; // increment counter by 1
} // end while
// termination phase
average = total / 10; // integer division yields integer result
// display total and average of grades
System.out.printf( "\nTotal of all 10 grades is %d\n", total );
System.out.printf( "Class average is %d\n", average );
} // end method determineClassAverage
}
C#
using System;
using System.Collections.Generic;
using System.Linq;
using System.Text;
using System.Threading.Tasks;
namespace Sample
{
class GradeBook
{
static void Main(string[] args)
{
DetermineClassAverage();
}
Unit 3 [IT213: Software Development Concepts]
public static void DetermineClassAverage()
{
int total; // sum of the grades entered by user
int gradeCounter; // number of the grade to be entered next
int grade; // grade value entered by the user
int average; // average of the grades
// initialization phase
total = 0; // initialize the total
gradeCounter = 1; // initialize the loop counter
// processing phase
while (gradeCounter <= 10) // loop 10 times
{
Console.Write("Enter grade: "); // prompt the user
grade = Convert.ToInt32(Console.ReadLine()); // read grade
total = total + grade; // add the grade to total
gradeCounter = gradeCounter + 1; // increment the counter by 1
} // end while
// termination phase
average = total / 10; // integer division yields integer result
// display total and average of grades
Console.WriteLine("\nTotal of all 10 grades is {0}", total);
Console.WriteLine("Class average is {0}", average);
Console.ReadKey();
} // end method DetermineClassAverage
} // end class GradeBook
} //End of Program
JavaScript
<html>
<head>
<meta charset = "utf-8">
<title>Class Average Program</title>
<h1>Class Average Program</h1>
</head>
<body>
Unit 3 [IT213: Software Development Concepts]
<p id="demo">Enter Grades</p>
<button type="button" onclick="myFunction()">Enter Grades</button>
<script>
//function myFunction() {
// document.getElementById("demo").innerHTML = "Paragraph changed.";
//}
function myFunction() {
//document.getElementById("demo").innerHTML = "Paragraph changed.";
var total; // sum of grades
var gradeCounther; // number of grades entered
var grade; // grade typed by user (as a string)
var gradeValue; // grade value (converted to integer)
var average; // average of all grades
//Unit 3 [IT213: Software Development Concepts]
// initialization phase
total = 0; // clear total
gradeCounter = 1; // prepare to loop
// processing phase
while ( gradeCounter <= 3 ) // loop 10 times
{
// prompt for input and read grade from user
grade = window.prompt("Enter integer grade:", "0" );
// convert grade from a string to an integer
Unit 3 [IT213: Software Development Concepts]
gradeValue = parseInt( grade );
// add gradeValue to total
total = total + gradeValue;
// add 1 to gradeCounter
gradeCounter = gradeCounter + 1;
document.writeln("<h1>Grade is " + gradeValue + "</h1>" );
//document.writeln("<h1>Grade is " + gradeValue + "</h1>" );
} // end while
// termination phase
average = total / 10; // calculate the average
// display average of exam grades
document.writeln("<h1>Class average is " + average + "</h1>" );
}
</script>
</body>
</html>
3-2. Write a program called SumAndAverage to produce the sum of the numbers 1 through 100. Use
a for loop for this program. Also, compute and display the average.
EXPECTED OUTPUT
The sum is 5050
The average is 50.5
3-3. Modify the code created for Exercise 3-2 to use a while loop instead of a for loop.
EXPECTED OUTPUT
The sum is 5050
Unit 3 [IT213: Software Development Concepts]
The average is 50.
Unit 3 Assignment: Coding Exercises
Exercise Criteria Possible Earned
Exercise 1 completed correctly 0-5
Exercise 2 completed correctly 0-5
Exercise 3 completed correctly 0-5
Total 0-15
Unit 3 Assignment 2: Coding Project
Using the language you have chosen to focus on: C#, Java, Web Development languages (PHP and
JavaScript), please complete the following two Assignments,
Using while-loops, create an example of a nested loop.
The outer loop will count from 5 to 1.
The inner loop will count from 0 to 10 by 2.
Use the variable k for the outer loop counter and the variable i for the inner loop counter.
Print out the index value for both k and i as the program executes through each loop.
Expected Output:
k = 5 i = 0
k = 5 i = 2
k = 5 i = 4
k = 5 i = 6
k = 5 i = 8
k = 5 i = 10
k = 4 i = 0
k = 4 i = 2
k = 4 i = 4
Unit 3 [IT213: Software Development Concepts]
k = 4 i = 6
k = 4 i = 8
k = 4 i = 10
k = 3 i = 0
k = 3 i = 2
k = 3 i = 4
k = 3 i = 6
k = 3 i = 8
k = 3 i = 10
k = 2 i = 0
k = 2 i = 2
k = 2 i = 4
k = 2 i = 6
k = 2 i = 8
k = 2 i = 10
k = 1 i = 0
k = 1 i = 2
k = 1 i = 4
k = 1 i = 6
k = 1 i = 8
k = 1 i = 10
Unit 3 Assignment 2: Coding Project Rubric
Criteria Points
Possible
Points
Earned
Unit 3 [IT213: Software Development Concepts]
Student names the variables correctly according to
Assignment instructions.
0-5
Student uses appropriate variable for the inner and outer
loop according to Assignment instructions.
0-5
Student uses appropriate count values for outer loop. 0-5
Student uses appropriate count values for inner loop. 0-5
Student correctly implements two while loops in a nested
loop format.
0-5
Program produces correct output. 0-5
Total 0-30
Unit 3 Assignment 3: Coding Project
Using a for-loop, write code that will countdown from 9 to 0, displaying the countdown number on the screen
with the associated count variable named “i”, and then print to the screen "These are the integers in the
decimal system!" after it prints the number 0.
Expected Output:
i= 9
i= 8
i= 7
i= 6
i= 5
i= 4
i= 3
i= 2
i= 1
Unit 3 [IT213: Software Development Concepts]
i = 0
These are the digits in the decimal system!
Unit 3 Assignment 3: Coding Project Rubric
Criteria Points Possible Earned
Student uses correct variable types. 0-5
Student correctly names the counter variable according to
Assignment instructions.
0-5
Student uses correct increment/decrement. 0-5
Student correctly implements a for loop.
Variable are declared correctly and correct values used.
Correct increment used.
0-5
0-5
Program produces correct output. 0-5
Total 0-30
[Solved] IT213 Unit 3 Assignment | Complete Solution
- This Solution has been Purchased 3 time
- Submitted On 03 Sep, 2017 11:33:52
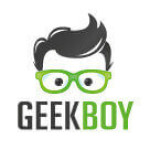
- ExpertT
- Rating : 109
- Grade : A+
- Questions : 1
- Solutions : 1026
- Blog : 0
- Earned : $53187.54
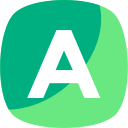
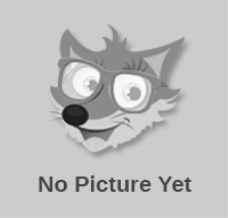
IT213 Unit 5 Assignment | Complete Solution
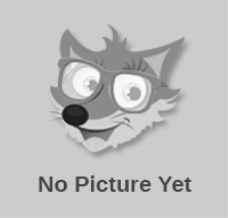
IT213 Unit 5 Assignment | Complete Solution
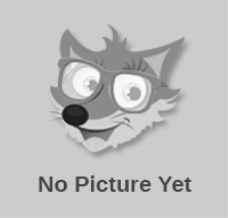
IT213 Unit 5 Assignment | Complete Solution
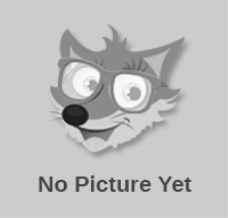
IT213 Unit 1 Assignment: Software Basics
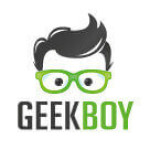
IT213 Unit 1 Assignment | Complete Solution
The benefits of buying study notes from CourseMerits
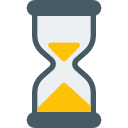
Assurance Of Timely Delivery
We value your patience, and to ensure you always receive your homework help within the promised time, our dedicated team of tutors begins their work as soon as the request arrives.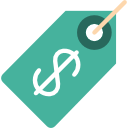
Best Price In The Market
All the services that are available on our page cost only a nominal amount of money. In fact, the prices are lower than the industry standards. You can always expect value for money from us.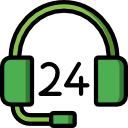