IT213 Unit 2 Assignment | Complete Solution
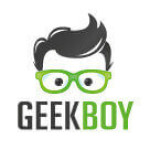
- ExpertT
- Rating : 109
- Grade : A+
- Questions : 1
- Solutions : 1026
- Blog : 0
- Earned : $53187.54
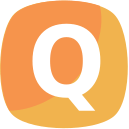
Unit 2 Assignment Instructions
In this unit you will complete the coding exercises before starting the coding Projects.
Review the detailed instructions and rubrics before starting your Assignments.
Submission Instructions
You will submit the following:
.cs files for C#
.java files for Java
.php and/or .js files for Web Development
Additionally, in a Word document, paste a screenshot of the output for each exercise.
You will be submitting three code files, one for each exercise (.cs, .java, .php or .js) and
one Word document in a zipped folder.
Zip these four files into a zipped folder and submit the one zipped folder.
Naming Your Files and Zip Folder
The code files should be saved as:
IT213_YourLastName_UnitX_ExerciseX_Language.
The word document should be saved as: IT213_YourLastName_UnitX_Screenshots
The zip folder should be saved as: IT213_YourLastName_UnitX_ZIP
Unit 2 Coding Exercises: Coding Exercises
You must complete the following coding exercises before starting the coding Projects.
By completing these exercises you will be better prepared for the Assignment.
Note: If your language of choice is Web Development, you will need to complete the
exercises in both PHP and JavaScript.
2-1.Type the following accurate code in the language of your choice. If there are errors, use the
accurate code to check against the code that you entered and fix any issues. Run the
completed code.
C#
1 // Fig. 3.18: Comparison.cs
2 // Comparing integers using if statements, equality operators
3 // and relational operators.
4 using System;
5
6 public class Comparison
7 {
8 // Main method begins execution of C# app
9 public static void Main( string[] args )
10 {
11 int number1; // declare first number to compare
12 int number2; // declare second number to compare
13
14 // prompt user and read first number
15 Console.Write( "Enter first integer: " );
16 number1 = Convert.ToInt32( Console.ReadLine() );
17
18 // prompt user and read second number
19 Console.Write( "Enter second integer: " );
20 number2 = Convert.ToInt32( Console.ReadLine() );
21
22 if ( number1 == number2
)
23 Console.WriteLine( "{0} == {1}", number1, number2
);
24
25 if ( number1 != number2
)
26 Console.WriteLine( "{0} != {1}", number1, number2
);
27
28 if ( number1 < number2
)
29 Console.WriteLine( "{0} < {1}", number1, number2
);
30
31 if ( number1 > number2
)
32 Console.WriteLine( "{0} > {1}", number1, number2
);
33
34 if ( number1 <= number2
)
35 Console.WriteLine( "{0} <= {1}", number1, number2
);
36
37 if ( number1 >= number2
)
38 Console.WriteLine( "{0} >= {1}", number1, number2
);
39 } // end Main
40 } // end class Comparison
Java
// Compare integers using if statements, relational operators
3 // and equality operators.
4 import java.util.Scanner; // program uses class Scanner
5
6 public class Comparison
7 {
8 // main method begins execution of Java application
9 public static void main( String[] args )
10 {
11 // create Scanner to obtain input from command line
12 Scanner input = new Scanner( System.in );
13
14 int number1; // first number to compare
15 int number2; // second number to compare
16
17 System.out.print( "Enter first integer: " ); // prompt
18 number1 = input.nextInt(); // read first number from user
19
20 System.out.print( "Enter second integer: " ); // prompt
21 number2 = input.nextInt(); // read second number from user
22
23 if ( number1 == number2 )
24 System.out.printf( "%d == %d\n", number1, number2 );
25
26 if ( number1 != number2 )
27 System.out.printf( "%d != %d\n", number1, number2 );
28
29 if ( number1 < number2 )
30 System.out.printf( "%d < %d\n", number1, number2 );
31
32 if ( number1 > number2 )
33 System.out.printf( "%d > %d\n", number1, number2 );
34
35 if ( number1 <= number2 )
36 System.out.printf( "%d <= %d\n", number1, number2 );
37
38 if ( number1 >= number2 )
39 System.out.printf( "%d >= %d\n", number1, number2 );
40 } // end method main
41 } // end class Comparison
JavaScript
<html>
<head>
<title>Exercise</title>
<script type="text/javascript">
function greaterNum(){
var value1 = document.getElementById('num1').value;
var value2 = document.getElementById('num2').value;
if (value1 > value2){
alert("Value 1 is greater than value 2");
Unit 2 [IT213: Software Development Concepts]
}
if (value2 > value1){
alert("Value 2 is greater than value 1");
}
if(value1 >= value2){
alert("Value 1 is equal to or greater than value 2")
}
if(value1 <= value2){
alert("Value 1 is equal to or less than value 2")
}
}
</script>
</head>
<body>
<form>
<label class="label1">Enter Value 1:</label>
<input type="number" name="num1" id = "num1">
<label class="label2">Enter Value 2:</label>
<input type="number" name="num2" id = "num2">
Unit 2 [IT213: Software Development Concepts]
<br/><br/>
<input type="button" value=" See relationship between numbers " onclick="greaterNum()">
</form>
</body>
</html>
2-2. Using the language of your choice (C#, Java, Web Development languages (PHP and JavaScript),
write code that represents the flowchart below.
Expected Output
Grade >= 60 Output will be Passed
Grade <60 Output will be nothing.
1-1. 2-3. Using the language of your choice (C#, Java, Web Development languages (PHP and JavaScript), write code
that represents the flowchart below.
Unit 2 [IT213: Software Development Concepts]
Grade >= 60 Output will be Passed
Grade <60 Output will be Failed
Unit 2 Assignment: Coding Exercises Rubric
Exercise Criteria Possible Earned
Exercise 1 completed correctly 0-5
Exercise 2 completed correctly 0-5
Exercise 3 completed correctly 0-5
Total 0-15
Unit 2 Assignment 2: Coding Project
Once you have completed the Unit 2 Coding Exercises, you can start working on the following Assignments:
Using the language you have chosen to focus on: C#, Java, Web Development languages (PHP and
JavaScript), please complete the following Assignment.
Use if, else if, and else statements to determine when a golf score is above, below or equal to par. Print the
message “score is below par” or “score is above par” or “score is equal to par” depending on your answer. Use
par and strokes as the variable names and set par=3 and strokes=4.
EXPECTED OUTPUT
The score is above par.
Unit 2 Assignment 2: Coding Project Rubric
Assignment Criteria Points Possible Earned
Program provides the correct/expected output. 0-5
Variables are correctly named according to Assignment instructions. 0-5
Variables are correctly typed. 0-5
Variables are initialized with the proper values according to the
instructions.
0-5
Conditionals are appropriately declared using the proper syntax.
Unit 2 [IT213: Software Development Concepts]
Uses if statement. 0-5
Uses else if statement. 0-5
TOTAL 0-30
Unit 2 Assignment 3: Coding Project
Write a switch statement to print out the full days of the week depending on a number 0-6, with 0
being ‘Sunday’. Use the variable selection Number and set it equal to 5 to execute the code.
EXPECTED OUTPUT
The day of the week is Friday
Unit 2 Assignment 3 Submission Instructions:
Review the How to Zip Project Folders in Visual Studio and Eclipse.
Submit the complete Project folder, zipped.
• For C# this will be the Visual Studio Project.
• For Java, PHP, and Javascript, this will be the Eclipse Project. .
File name format: IT213_YourLastName_UnitXProject_Language
Unit 2 Assignment 3: Coding Project Rubric
Assignment Criteria Points Possible Points Earned
Program provides the correct/expected output. 0-5
Variables are given the proper names according to
Assignment instructions.
0-5
Variables are correctly typed. 0-5
Switch statement is appropriately implemented
using the proper syntax.
Checks correct variable
0-5
Case statements implemented correctly. 0-5
Input variable is assigned the proper value
according to the assignment instructions.
0-5
Unit 2 [IT213: Software Development Concepts]
Total 0-30
[Solved] IT213 Unit 2 Assignment | Complete Solution
- This Solution has been Purchased 4 time
- Submitted On 03 Sep, 2017 11:15:41
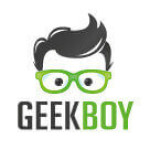
- ExpertT
- Rating : 109
- Grade : A+
- Questions : 1
- Solutions : 1026
- Blog : 0
- Earned : $53187.54
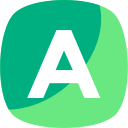
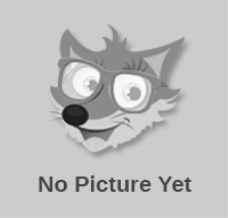
IT213 Unit 5 Assignment | Complete Solution
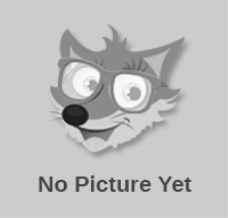
IT213 Unit 5 Assignment | Complete Solution
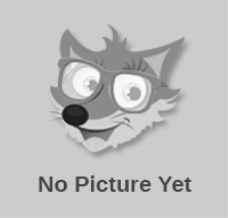
IT213 Unit 5 Assignment | Complete Solution
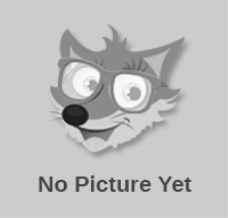
IT213 Unit 1 Assignment: Software Basics
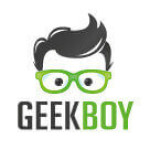
IT213 Unit 1 Assignment | Complete Solution
The benefits of buying study notes from CourseMerits
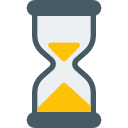
Assurance Of Timely Delivery
We value your patience, and to ensure you always receive your homework help within the promised time, our dedicated team of tutors begins their work as soon as the request arrives.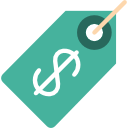
Best Price In The Market
All the services that are available on our page cost only a nominal amount of money. In fact, the prices are lower than the industry standards. You can always expect value for money from us.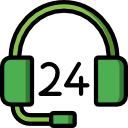