COMP122 Week 7 iLab | Complete Solution
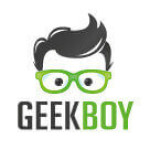
- ExpertT
- Rating : 109
- Grade : A+
- Questions : 1
- Solutions : 1026
- Blog : 0
- Earned : $53187.54
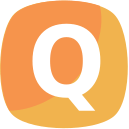
COMP122 Week 7 iLab
The focus of this lab is on using strings. You willhave an opportunity to work with both C style strings and the string data type. This lab also givesyou an opportunity to use what you have learned previously, including usingfunctions, array processing, repetition, and selection. You will also have anopportunity to work with file input and output.
You are to design and implement a program which doesencryption and decryption of data from files. Encryption is the process oftaking plain lines of text and performing some algorithmic transformation onthe data to create an encrypted line of text which looks nothing like theoriginal. Decryption is the process of taking an encrypted line of text andperforming some algorithmic transformation on the data to recover the originalline of plain text.
Encryptionand Decryption Approach
Our approach to encryption and decryption involvestwo strings. The first is an encryption / decryption string which we will allowto be up to 128 lower case alphabetical characters in length. The second stringis a line of text from a file that is to be encrypted or decrypted.
Our basic strategy for encrypting data is based onmapping alphabetical characters to specific values, then doing some simplemathematical operations to create a new value. First of all, every character ineither the encryption string or the input string is mapped to a number between0 and 25 based on its position in the alphabet.
A =a = 0
B = b = 1
Z = z = 25
The mapped value of a character is easily obtainedby doing the following:
For lower casecharacters, subtract 'a' from the character.
For upper case characters, subtract 'A' from the character.
To calculate the modified value of the firstcharacter of input we add its mapped value to the mapped value from the firstcharacter of the encryption string. This modified value is then adjusted using% 26 to make sure that the final modified value is within the 0 - 25 range. Tocreate the final encrypted character value for the first character, simply dothe following:
For lower casecharacters, add 'a' to the modified value.
For upper case characters, add 'A' to the modified value.
This is done for each alphabetic character in theinput string. Non-alphabetic characters simply maintain their present value. Ifthe input string is longer than the encryption string, simply reuse mappedvalues from the encryption string. For instance, if the encryption string has10 characters (index values 0 - 9), when processing the 11th input character (index 10), simply use theinput character index % length of encryption string (in this case 10 % 10 is 0)to select the value from the encryption string to use for mapping.
The decryption process is basically the same as theencryption process. The only difference is the value of the mapped characterfrom the encryption string.
For lower case encryption, the mapped value =character from encryption string - 'a'
For upper case encryption, the mapped value = character from encryption string -'A'
For lower case decryption, the mapped value = 26 -(character from encryption string - 'a')
For upper case decryption, the mapped value = 26 - (character from encryptionstring - 'A')
ProgramRequirements
Your program must meet the following requirements:
1. You must ask the user if they want to perform anencryption or decryption operation.
2. You must ask the user to enter the name of the file they want to encrypt ordecrypt.
3. You must get an encryption key from the user which can be up to 128characters. The key must be all lower case alphabetic characters.
4. You must have a function whichtakes the encryption key and creates an encryption map from it. For eachcharacter in the encryption key string, subtract the lower case letter 'a' andstore the result in the corresponding encryption map array.
5. You must have a function whichtakes the encryption key and creates a decryption map from it. For eachcharacter in the encryption key string, subtract the lower case letter 'a' fromit. Then subtract that result from 26 and store the value in the correspondingdecryption map array.
6. You must have a function whichwill do the encryption or decryption transformation. This function takes thefollowing parameters:
A constant C string containing the line of text to be transformed.
A constant C character arraywhich contains the encryption or decryption map.
An integer which contains thelength of the encryption map.
A string reference (output) which will contain the encrypted ordecrypted string upon completion.
The core of the encryption / decryption algorithm isas follows:
Foreach character (the ith character) in the text input line do the following:
if the characteris not alphabetical, add it to the end of the output string
if the characteris lower case alphabetical
subtractthe character 'a' from the character
getthe ith % map length element from the map and add it to the character
adjustthe value of the character % 26 to keep it within the alphabet
addthe character 'a' to the character
addthe encrypted character value to the end of the output string
if the characteris upper case alphabetical
do thesame thing as for lower case except use 'A' instead of 'a'
7. For decryption, the main program should create anifstream for the file to be decrypted. It should use the getline method of the ifstream to read lines from the file, callthe encryption / decryption function with the line to be decrypted, and displaythe string which contains the result of the encryption / decryption functioncall. Repeat until the ifstream reaches the end of the file, then close theifstream.
8. For encryption, the main program should create anifstream for the file to be encrypted. It should also create an ofstream forthe file where the encrypted result will be stored. The file name for this filecan be gotten from the user or can be the input file name with a specialextension added at the end. The getlinemethod of the ifstream is used to read lines from the input file. Then theencryption / decryption function is called to encrypt the line. Display thestring containing the result and write the string to the ofstream. Close theifstream and ofstreams when finished.
9. Make sure that your program allows the user toencrypt / decrypt more than one file per session. This means adding a loopwhich allows the entire program to repeat until the user has nothing more todo.
Hints
1. UseC strings for the encryption string and the file names. Use char arrays for theencryption and decryption maps. You cannot treat these as C strings because themaps can contain 0 as a valid data item rather than the end of string marker.
2. Usea string type variable to hold the encrypted and decrypted strings. The stringtype allows you to add characters to theend of a string using the push_backmethod, and it allows you to dump thecontents of the string using the erasemethod.
3.For input streams, you can use the eofmethod to determine when you have reached the end of a file.
4.Use a character array to read data from the files. Set the maximum length forthis buffer to be 256 characters.
DevelopmentStrategy
I would recommend that you build this project in twophases. The first phase should concentrate on getting the encryption anddecryption map functions and the encryption / decryption function working. Youcan test this by using fixed C strings for the input line and the encryptionstring. Call the map functions, then encrypt the fixed input string, output theresult, then decrypt the encrypted string and output the result. When yourfinal output is the same as the original input, your encryption / decryptionfunctions are working. The second phase adds the file operations.
Testingand Deliverables
When you think you have a working program, use Notepadto create a file with plain text in it. You should enter some different lengthlines containing a variety of characters. Your file should have at least 10lines. You should try using short and long encryption keys. Using your program,encrypt the file, then decrypt the encrypted file. Take a screen shot of yourdecrypted output and paste it into a Word document. Also copy the contents ofyour original file and the encrypted file into the Word document. Clearly labelthe contents of the Word document. Then copy your source code into yourdocument. Make sure that you have usedproper coding style and commenting conventions!
[Solved] COMP122 Week 7 iLab | Complete Solution
- This solution is not purchased yet.
- Submitted On 17 Apr, 2015 11:33:08
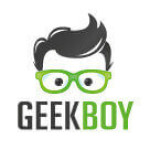
- ExpertT
- Rating : 109
- Grade : A+
- Questions : 1
- Solutions : 1026
- Blog : 0
- Earned : $53187.54
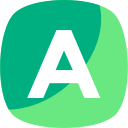
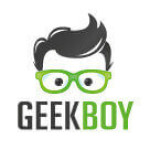
COMP122 Week 7 iLab | Complete Solution
The benefits of buying study notes from CourseMerits
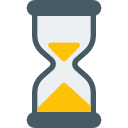
Assurance Of Timely Delivery
We value your patience, and to ensure you always receive your homework help within the promised time, our dedicated team of tutors begins their work as soon as the request arrives.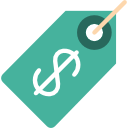
Best Price In The Market
All the services that are available on our page cost only a nominal amount of money. In fact, the prices are lower than the industry standards. You can always expect value for money from us.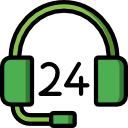