CSE 110 - Lab 9 complete solutions correct answers key
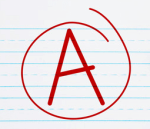
- Vpqnrqhwk
- Rating : 40
- Grade : A+
- Questions : 2
- Solutions : 1079
- Blog : 0
- Earned : $19352.58
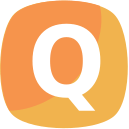
CSE 110 - Lab 9 complete solutions correct answers key
What this Lab Is About:
More practice of:
• Classes and objects
• Constructors
• getter methods that return a value
• setter methods that take arguments and set the state of an object
Use the following Coding Guidelines:
• When declaring a variable, you usually want to initialize it.
• Remember you cannot initialize a number with a string.
• Remember variable names are case sensitive.
• Use tabs or spaces to indent code within blocks (code surrounded by braces). This includes classes, methods, and code associated with ifs, switches and loops. Be consistent with the number of spaces or tabs that you use to indent.
• Use white space to make your program more readable.
• Use comments after the ending brace of classes, methods, and blocks to identify to which block it belongs.
Assignments Documentation:
At the beginning of each programming assignment you must have a comment block with the following information:
/*-------------------------------------------------------------------------
// AUTHOR: your name
// FILENAME: Lab9.java
// SPECIFICATION:
// FOR: CSE 110- Lab #9
// TIME SPENT: how long it took you to complete the assignment
//----------------------------------------------------------------------*/
Problem Description: Expand the Student Class
For this Lab your task is to create a class Employee. Employee class should have the following instance variables: empID, empName, empSalary, emplJob,empRating that store the employee’s ID, name, salary, job title, and number indicating the performance of the employee respectively. You should also supply a constructor and the following methods: getempID(), getempSalary(),getName(), setempSalary(), setempJob(), raiseempSalary().
Step 1: Getting Started:
First, you need to create an Employee class and declare class as follows
class Employee {
Inside the class Employee, you will be declaring the following data members to store employees’ information: a String variable empName, an integer empID, a double empSalary, a String empJob and an int empYears.
// declare some variables of different types:
// a String called empName
//-->
// an int called empID
//-->
// a String called empJob
//-->
// a double called empSalary
//-->
// an int called empRating
//-->
Step 3: Defining the constructor
public Employee (String employeeName, int employeeID, int employeeRating)
{
// write the segment of code
//that assigns input data to the data members
}
Step 4: Adding the methods
You will need to create the following getter and setter methods:
int getempID()
String getName() double getempSalary()
void setempSalary(double salary)
void setempJob(String jobTitle)
These getter and setter methods are quite similar to the ones we created for Lab 7 and Lab 8. Refer to the Lab 7 and Lab 8 handout for the creation and function of getter and setter methods.
In addition to the getter and setter methods, you will create an additional method that raises the salary of the employee based on the performance of the employee. Here is the logic to implement the raiseSalary() class:
If the number indicating the performance of the employee is 1, employee will receive a raise of 4%.
If the number indicating the performance of the employee is 2, employee will receive a raise of 2%.
If the number indicating the performance of the employee is 3, employee will not receive a raise.
void raiseSalary() {
//finish implementing the raiseSalary method
if (empRating==1){
empSalary= empSalary+ (empSalary*0.04)
}
-->
}
Step 5: Calling the constructor and using the methods of the Employee class from the “main” method
The final task of this lab is use Employee class variables and methods. In order to do so, we need to have a runner function. Remember, for any class in java, “main” method will be the running method.
public class Lab9 {
public static void main(String[] args) {
// Create a new Scanner object to take in input from the user.
// ->
// Prompt the user for the employee’s name
// ->
// Prompt the user for the employee’s ID
// ->
// Prompt the user for employee’s rating.
// ->
// create a new Employee object using the name,
// id, job, salary, and rating given by the user
//-->
//Since we did not prompt for job title
// use the setempJob(String jobTitle) to set the job to
//Software Engineer
// -->
//Since we did not prompt for employee’s salary
// use the setempSalary(double salary) to set the salary to
//4000.00
// ->
// Print out the Employee’s information in the
// following format:
// Employee’s Information:
// ->
// The employee’s full name is: <Employee Name>
// ->
// The employee’s ID number is: <Employee ID>
// ->
// The employee’s current salary is: <Employee salary>
// ->
// Attempt to check if employee’s is eligible for a salary raise
// Apply the raiseSalary() method to the employee’s object
// -->
// Print out the following:
// <Employee’s Name> new salary is <Employee Salary>
-->
}
}
Sample Output
Below is an example of what your output should roughly look like when this lab is completed.
Text in green represents user input.
Enter employee's name:
Jack Mann
Enter employee's Id:
123456789
Enter employee's rating:
1
Employee's Information
The employee’s full name is: Jack Mann
The employee’s ID number is: 123456789
The employee’s current salary is: 4000.0
Jack Mann new salary is y is: 4160.0
Enter employee's name:
Bill John
Enter employee's Id:
98762345
Enter employee's rating:
3
Employee's Information
The employee’s full name is: Bill John
The employee’s ID number is: 98762345
The employee’s current salary is: 4000.0
Bill John new salary is y is: 4000.0
Submission:
Submit your Lab9.java file to the Submission Server. Go to the Submission Server site,
https://courses.eas.asu.edu/cse110b/ login, then click on Lab Submissions in the left frame.
Choose Lab9 from the dropdown box, click on the browse button and find where you saved your Lab9.java file (and not the Lab9.class file) on your computer. Upload the file to the site and then click on the Submit button.
Your file will be submitted and a screen will show up displaying if your program compiled and what your output is when run on some sample input.
You should then check to make sure that the actual file submitted properly and is readable to the grader. To do so, click on Grades in the frame on the left of the page and then click on the 0 underneath Lab9. You will again see that your program compiled and the sample output, but you should scroll down to the bottom of the screen and make sure your file is readable as well.
Important Note: You may resubmit as many times as you like until the deadline, but we will only mark your last submission.
[Solved] CSE 110 - Lab 9 complete solutions correct answers key
- This solution is not purchased yet.
- Submitted On 12 Jan, 2017 03:12:12
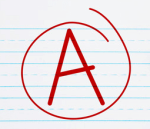
- Vpqnrqhwk
- Rating : 40
- Grade : A+
- Questions : 2
- Solutions : 1079
- Blog : 0
- Earned : $19352.58
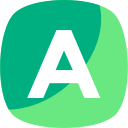
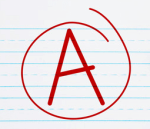
CSE 110 - ASSIGNMENT # 7 – complete solutions correct answers key
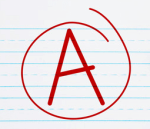
CSE 110 - Lab 9 complete solutions correct answers key
The benefits of buying study notes from CourseMerits
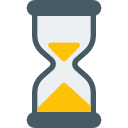
Assurance Of Timely Delivery
We value your patience, and to ensure you always receive your homework help within the promised time, our dedicated team of tutors begins their work as soon as the request arrives.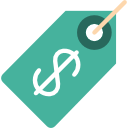
Best Price In The Market
All the services that are available on our page cost only a nominal amount of money. In fact, the prices are lower than the industry standards. You can always expect value for money from us.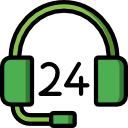