CSE205 Object Oriented Programming and Data Structures Homework 2 + SUBMISSION
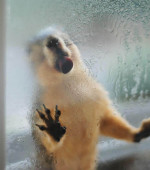
- Halsey
- Rating : 15
- Grade : A+
- Questions : 0
- Solutions : 335
- Blog : 0
- Earned : $5956.25
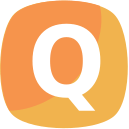
CSE205 Object Oriented Programming and Data Structures Homework 2 :: 25 pts
1 Submission Instructions
Create a document using your favorite word processor and type your exercise solutions. At the top of the document be
sure to include your name and the homework assignment number, e.g. HW2. Convert this document into Adobe PDF
format and name the PDF file .pdf where is your ASURITE user id (for example, my ASURITE
user id is kburger2 so my file would be named kburger2.pdf). To convert your document into PDF format, Microsoft Office
versions 2008 and newer will export the document into PDF format by selecting the proper menu item from the File
menu. The same is true of Open Office and Libre Office. Otherwise, you may use a freeware PDF converter program, e.g.,
CutePDF is one such program.
Next, create a folder named and copy .pdf to that folder. Copy any Java source code files to this
folder (note: Java source code files are the files with a .java file name extension; do not copy the .class files as we do not
need those).
Next, compress the folder creating a zip archive file named .zip. Upload .zip to the
Homework Assignment 2 dropbox by the assignment deadline. The deadline is 11:59pm Wed 9 Apr. Consult the online
syllabus for the late and academic integrity policies.
Note: not all of these exercises will be graded, i.e., random ones will be selected for grading.
2 Learning Objectives
1. Properly use the public, private, and protected accessibility modifiers.
2. Write Java code to override and overload methods.
3. Recognize when inheritance is present among classes in an OOD.
4. Implement classes using inheritance.
5. To recognize when polymorphism is present in an inheritance hierarchy and to implement it.
6. To declare and implement a Java interface.
7. To implement a GUI.
3 Objects, Classes, and Inheritance
3.1 Is it required to provide an accessor and/or mutator method for every instance variable of a class? If yes, explain
why this is required, and if no, explain why not.
3.2 Suppose the class Sub extends Sandwich. Which of the following statements are legal?
Sandwich x = new Sandwich();
Sub y = new Sub();
(a) x = y;
(b) y = x;
(c) Sub y = new Sandwich();
(d) Sandwich x = new Sub();
3.3 True or False? A subclass declaration will generally contain declarations for instance variables that are specific to
object of that subclass, i.e., those instance variables represent attributes that are not part of superclass objects.
3.4 True or False? A superclass declaration will generally contain declarations for instance variables that are specific to
objects of that superclass, i.e., those instance variables represent attributes that are not part of subclass objects.
Arizona State University Page 1
CSE205 Object Oriented Programming and Data Structures Homework 2 :: 25 pts
3.5 Consider classes C1, C2, and C3. Answer the following questions.
class C1 {
public int x1;
protected int x2;
private int x3;
public void c1Method1() {}
protected void c1Method2() {}
private void c1Method3() {}
}
class C2 extends C1 {
public int y1;
protected int y2;
private int y3;
public void c2Method1() {}
protected void c2Method2() {}
private void c2Method3() {}
}
class C3 extends C2 {
public int z1;
protected int z2;
private int z3;
public void c3Method1() {}
protected void c3Method2() {}
private void c3Method3() {}
}
1. Which instance variables x1, x2, x3 declared in C1 are directly accessible in c1Method1()?
2. Which instance variables x1, x2, x3 declared in C1 are directly accessible in c1Method2()?
3. Which instance variables x1, x2, x3 declared in C1 are directly accessible in c1Method3()?
4. Which instance variables x1, x2, x3 declared in C1 are directly accessible in c2Method1()?
5. Which instance variables x1, x2, x3 declared in C1 are directly accessible in c2Method2()?
6. Which instance variables x1, x2, x3 declared in C1 are directly accessible in c2Method3()?
7. Which instance variables x1, x2, x3 declared in C1 are directly accessible in c3Method1()?
8. Which instance variables x1, x2, x3 declared in C1 are directly accessible in c3Method2()?
9. Which instance variables x1, x2, x3 declared in C1 are directly accessible in c3Method3()?
10. Which instance variables y1, y2, y3 declared in C2 are directly accessible in c1Method1()?
11. Which instance variables y1, y2, y3 declared in C2 are directly accessible in c1Method2()?
12. Which instance variables y1, y2, y3 declared in C2 are directly accessible in c1Method3()?
13. Which instance variables z1, z2, z3 declared in C3 are directly accessible in c1Method1()?
14. Which instance variables z1, z2, z3 declared in C3 are directly accessible in c1Method2()?
15. Which instance variables z1, z2, z3 declared in C3 are directly accessible in c1Method3()?
16. Which instance methods c1Method1(), c1Method2(), c1Method3() are callable from c2Method1()?
17. Which instance methods c1Method1(), c1Method2(), c1Method3() are callable from c2Method2()?
18. Which instance methods c1Method1(), c1Method2(), c1Method3() are callable from c2Method3()?
19. Which instance methods c1Method1(), c1Method2(), c1Method3() are callable from c3Method1()?
20. Which instance methods c1Method1(), c1Method2(), c1Method3() are callable from c3Method2()?
21. Which instance methods c1Method1(), c1Method2(), c1Method3() are callable from c3Method3()?
22. Which instance methods c2Method1(), c2Method2(), c2Method3() are callable from c1Method1()?
23. Which instance methods c2Method1(), c2Method2(), c2Method3() are callable from c1Method2()?
24. Which instance methods c2Method1(), c2Method2(), c2Method3() are callable from c1Method3()?
25. How many instance variables are encapsulated within a C1 object?
26. How many instance variables are encapsulated within a C2 object?
27. How many instance variables are encapsulated within a C3 object?
3.6 Explain what an overloaded method is and give an example.
3.7 Explain what an overridden method is and give an example.
3.8 Explain what accidental overloading is and the preferred Java method for preventing it.
3.9 If an overridden method in a subclass needs to call the overridden superclass method, how is this accomplished?
3.10 True or False? It is legal for a method in a class to overload another method also in the same class. Explain.
3.11 True or False? It is legal in a class for a method to override another method also in the same class. Explain.
3.12 True or False? It is legal in a subclass for a method to overload a method in the superclass. Explain.
3.13 True or False? It is legal in a subclass for a method to override a method in the superclass. Explain.
Arizona State University Page 2
CSE205 Object Oriented Programming and Data Structures Homework 2 :: 25 pts
3.14 True or False? It is legal in a superclass for a method to overload a method in a subclass. Explain.
3.15 True or False? It is legal in a superclass for a method to override a method in a subclass. Explain.
3.16 In a subclass constructor, the superclass default constructor is called automatically before the statements of the
subclass constructor begin executing. Suppose we wish to call a different superclass constructor (i.e., not the default
constructor) from the subclass constructor. Explain how this is accomplished and give an example.
3.17 Explain how an abstract class differs from a concrete class.
4 Objects, Classes, Polymorphism, and Interfaces
4.1 In the video lecture for Interfaces : Section 6 we discussed an example program that implements an inheritance
hierarchy (Mammal is the superclass of Cat and Dog; Insect is the superclass of Cricket). Which method or methods
in that program are called polymorphically?
4.2 Write the Java code to declare a new class Bee which is a subclass of Insect. The noise made by a Bee is "Buzz".
4.3 Write the Java code to declare a new abstract class Amphibian that implements the MakesNoise interface.
4.4 Write the Java code to declare a new class Frog which is a subclass of Amphibian. The noise made by a Frog is
"Ribbet".
4.5 Modify the run() method of Main and add some Bees and Frogs to critters. Build your program and verify that it
works correctly. Include all of your .java source code files in the zip archive that you submit for grading.
5 GUI Programming
5.1 For these exercises, include your completed .java files in the zip archive that you submit for grading. Complete the
code in the provided View class to implement this GUI interface for a calculator. The calculator does not have to be
fully functional; the primary objective of the assignment is to implement the GUI.
5.2 Complete the code in actionPerformed() so when the Exit button is clicked, the application will terminate.
5.3 Complete the code in actionPerformed() so when the About button is clicked, the application will display this about
dialog:
Arizona State University Page 3
CSE205 Object Oriented Programming and Data Structures Homework 2 :: 25 pts
6 Nested Classes
6.1 Explain what an inner class is.
6.2 Explain how a local class differs from an inner class.
6.3 Explain how an anonymous class differs from an inner and local class.
Arizona State University Page 4
CSE205 Object Oriented Programming and Data Structures Programming Project 2 :: 25 pts
1 Submission Instructions
Create a folder named <asuriteid> where asuriteid is your ASURITE user id (for example, since my ASURITE user id is
kburger2 my folder would be named kburger2) and copy all of your .java source code files to this folder. Do not copy the
.class files or any other files. Next, compress the <asuriteid> folder creating a zip archive file named <asuriteid>.zip
(mine would be named kburger2.zip). Upload <asuriteid>.zip to the Project 2 dropbox by the project deadline. The
deadline is 11:59pm Wed 9 Apr. Consult the online syllabus for the late and academic integrity policies.
2 Learning Objectives
1. Read UML class diagrams and convert the diagram into Java classes.
2. Identify and implement dependency, aggregation, inheritance, and composition relationships.
3. Properly use the public, private, and protected accessibility modifiers.
4. Write Java code to override methods.
5. Recognize when inheritance is present among classes in an OOD.
6. Design and implement classes using inheritance.
7. To write Java code to implement polymorphism in a class inheritance hierarchy.
8. To implement a Java interface.
3 Background
At Springfield State U there are two classes of students: on-campus students and online students. On-campus students are
categorized as residents (R) or nonresidents (N) depending on whether they reside within the state in which Springfield
exists or they reside in a different state. The base tuition for on-campus students is $5500 for residents and $12,200 for
non-residents. Some on-campus students, enrolled in certain pre-professional programs, are charged an additional program
fee which varies depending on the program. An on-campus students may enroll for up to 18 credit hours at the base rate
but for each credit hour exceeding 18, they pay an additional fee of $350 for each credit hour over 18.
Online students are neither residents nor non-residents. Rather, their tuition is computed as the number of credit hours
for which they are enrolled multiplied by the online credit hour rate which is $875 per credit hours. Furthermore, some
online students enrolled in certain degree programs pay an online technology fee of $125 per semester.
4 Software Requirements
Your program shall meet these requirements.
1. Student information for Springfield State University is stored in a text file named p02-students.txt. There is one
student record per line, where the format of a student record for an on-campus student is:
C id last-name first-name residency program-fee credits
where:
'C' Identifies the student as an on-campus student.
id The student identifier number. A string of 13 digits.
last-name The student's last name. A contiguous string of characters.
first-name The student's first name. A contiguous string of characters.
residency 'R' if the student is a resident, 'N' if the student is a non-resident.
program-fee A program fee, which may be zero.
credits The number of credit hours for which the student is enrolled.
The format of a student record for an online student is:
O id last-name first-name tech-fee credits
where 'O' identifies the student as an online student, and id, last-name, first-name, and credits are the same as
for an on-campus student. The tech-fee field is 'T' if the student is to be assessed the technology fee or '-' if the
student is not assessed the technology fee.
Arizona State University Page 1
CSE205 Object Oriented Programming and Data Structures Programming Project 2 :: 25 pts
Here is an example p02-students.txt file:
Sample p02-students.txt
C 8230123345450 Flintstone Fred R 0 12
C 3873472785863 Simpson Lisa N 750 18
C 4834324308675 Jetson George R 0 20
O 1384349045225 Szyslak Moe - 6
O 5627238253456 Flanders Ned T 3
2. The program shall read the contents of p02-students.txt and calculate the tuition for each student.
3. The program shall write the tuition results to an output file named p02-tuition.txt formatted thusly:
id last-name first-name tuition
id last-name first-name tuition
...
where tuition is the computed tuition for the student. The tuition shall be displayed with two digits after the
decimal point. For example:
Sample p02-tuition.txt
1384349045225 Szyslak Moe 5250.00
3873472785863 Simpson Lisa 12950.00
4834324308675 Jetson George 6200.00
5627238253456 Flanders Ned 2750.00
8230123345450 Flintstone Fred 5500.00
4. The records in the output file shall be sorted in ascending order by id.
5. If the input file p02-students.txt cannot be opened for reading (because it does not exist) then display an error
message on the output window and immediately terminate the program, e.g.,
run program
Sorry, could not open 'p02-students.txt' for reading. Stopping.
5 Software Design
Refer to the UML class diagram in Section 5.7. Your program shall implement this design.
5.1 Main Class
A template for Main is included in the zip archive. The Main class shall contain the main() method which shall
instantiate an object of the Main class and call run() on that object. Complete the code by reading the comments and
implementing the pseudocode.
5.2 TuitionConstants Class
The complete TuitionConstants class is included in the zip archive. This class simply declares some public static constants
that are used in other classes.
5.3 Sorter Class
We shall discuss sorting later in the course, so this code may not make perfect sense at this time. However, I have
provided all of it for you.
The Sorter class contains a public method insertionSort() that can be called to sort a list of ArrayList<Student>. When
sorting Students we need to be able to compare one Student A to another Student B to determine if A is less than or
greater than B. Since we are sorting by student id, we have the abstract Student class implement the Comparable
<Student> interface and we define Student A to be less than Student B if the mId field of A is less than the mId field of
B. This is how we sort the ArrayList<Student> list by student id.
Arizona State University Page 2
CSE205 Object Oriented Programming and Data Structures Programming Project 2 :: 25 pts
java.lang.Comparable<T> is a generic interface (it requires a type parameter T to be specified when the interface is
implemented) in the Java Class Library that declares one method:
int compareTo(T obj)
where T represents a class type and obj is an object of the class T. The method returns a negative integer if this T (the
object on which the method is invoked) is less than obj, zero if this T and obj are equal, or a positive integer if this T is
greater than obj. To make Student implement the Comparable interface, we write:
public abstract class Student implements Comparable<Student> { ... }
Since Student implements Comparable<Student>, whenever compareTo() is called in Sorter.keepMoving() to compare two
objects, either OnCampusStudent.compareTo() or OnlineStudent.compareTo() will be called.
5.4 Student Class
The Student class is an abstract class that implements the java.lang.Comparable interface (see 5.3 Sorter Class):
public abstract class Student implements Comparable<Student> {
...
}
A Student object contains five instance variables:
mCredits Number of credit hours the student is enrolled for.
mFname The student's first name.
mId The student's id number.
mLname The student's last name.
mTuititon The student's computed tuition.
Most of the Student instance methods should be straightforward to implement so we will only mention the two that are
not so obvious:
+calcTuition(): void
An abstract method that is implemented by subclasses of Student. Abstract methods do not have to be implements, and
this one is not.
+compareTo(pStudent: Student): int <<override>>
Implements the compareTo() method of the Comparable<Student> interface. Returns -1 if the mId instance variable of
this Student is less than the mId instance variable of pStudent. Returns 0 if they are equal (should not happen because id
numbers are unique). Returns 1 if the mId instance variable of this Student is greater than the mId instance variable of
pStudent. The code is for compareTo() is:
return getId().compareTo(pStudent.getId());
5.5 OnCampusStudent Class
The OnCampusStudent class is a direct subclass of Student. It adds new instance variables that are specific to on-campus
students:
mResident True if the OnCampusStudent is a resident, false for non-resident.
mProgramFee Certain OnCampusStudent's pay an additional program fee. This value may be 0.
The OnCampusStudent instance methods are mostly straightforward to implement so we shall only discuss two of them.
+OnCampusStudent(pId: String, pFname: String, pLname: String): <<ctor>>
Must call the superclass constructor passing pId, pFname, and pLname as parameters.
Arizona State University Page 3
CSE205 Object Oriented Programming and Data Structures Programming Project 2 :: 25 pts
+calcTuition(): void <<override>>
Must implement the rules described in Section 3 Background. Note that we cannot directly access the mTuition instance
variable of an OnCampus Student because it is declared as private in Student. So how do we write to mTuition? By calling
the protected setTuition() method that is inherited from Student. The pseudocode for calcTuition() is:
Override Method calcTuititon() Returns Nothing
Declare double variable t
If getResidency() returns true Then
t = TuitionConstants.ONCAMP_RES_BASE
Else
t = TuitionConstants.ONCAMP_NONRES_BASE
End if
t = t + getProgramFee();
If getCredits() > TuitionConstants.MAX_CREDITS Then
t = t + (getCredits() - TuitionConstants.MAX_CREDITS) × TuitionConstants.ONCAMP_ADD_CREDITS
End if
Call setTuition(t)
End Method calcTuition()
5.6 OnlineStudent Class
The OnlineStudent class is a direct subclass of Student. It adds a new instance variable that is specific to online students:
mTechFee Certain OnlineStudent's pay an additional technology fee. This instance variable will be true if the
technology fee applies and false if it does not.
The OnlineStudent instance methods are mostly straightforward to implement so we shall only discuss two of them.
+OnlineStudent(pId: String, pFname: String, pLname: String): <<ctor>>
Must call the superclass constructor passing pId, pFname, and pLname as parameters.
+calcTuition(): void <<override>>
Must implement the rules described in Section 3 Background. Note that we cannot directly access the mTuition instance
variable of an OnlineStudent because it is declared as private in Student. So how do we write to mTuition? By calling the
protected setTuition() method that is inherited from Student. The pseudocode for calcTuition() is:
Override Method calcTuititon() Returns Nothing
Declare double variable t = getCredits() × TuitionConstants.ONLINE_CREDIT_RATE
If getTechFee() returns true Then
t = t + TuitionConstants.ONLINE_TECH_FEE
End if
Call setTuition(t)
End Method calcTuition()
Arizona State University Page 4
CSE205 Object Oriented Programming and Data Structures Programming Project 2 :: 25 pts
5.7 UML Class Diagram
The UML class diagram shown below was created using UMLet. See the zip archive for the UMLet file. We have these
relationships:
Arizona State University Page 5
CSE205 Object Oriented Programming and Data Structures Programming Project 2 :: 25 pts
6 Additional Project Requirements
1. Format your code neatly. Use proper indentation and spacing. Study the examples in the book and the examples the
instructor presents in the lectures and posts on the course website.
2. Put a comment header block at the top of each method formatted thusly:
/**
* A brief description of what the method does.
*/
3. Put a comment header block at the top of each source code file formatted thusly:
//********************************************************************************************************
// CLASS: classname (classname.java)
//
// DESCRIPTION
// A description of the contents of this file.
//
// COURSE AND PROJECT INFO
// CSE205 Object Oriented Programming and Data Structures, semester and year
// Project Number: project-number
//
// AUTHOR
// your-name (your-email-addr)
//********************************************************************************************************
Arizona State University Page 6
[Solved] CSE205 Object Oriented Programming and Data Structures Homework 2 + SUBMISSION
- This Solution has been Purchased 13 time
- Submitted On 03 Nov, 2016 07:36:22
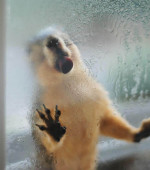
- Halsey
- Rating : 15
- Grade : A+
- Questions : 0
- Solutions : 335
- Blog : 0
- Earned : $5956.25
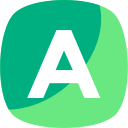
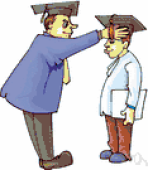
CSE205 Object Oriented Programming and Data Structures Homework 4 :
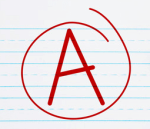
CSE205 Object Oriented Programming and Data Structures Homework 2 complete solutions correct answers
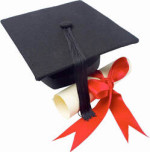
CSE205 Object Oriented Programming and Data Structures Homework 4
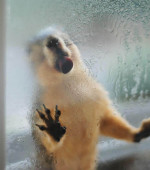
CSE205 Object Oriented Programming and Data Structures Homework 2 + SUBMISSION
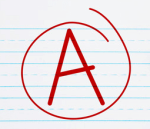
CSE205 Object Oriented and Data Structures Project 3 complete solutions correct answers key
The benefits of buying study notes from CourseMerits
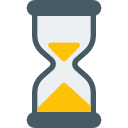
Assurance Of Timely Delivery
We value your patience, and to ensure you always receive your homework help within the promised time, our dedicated team of tutors begins their work as soon as the request arrives.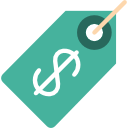
Best Price In The Market
All the services that are available on our page cost only a nominal amount of money. In fact, the prices are lower than the industry standards. You can always expect value for money from us.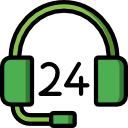