Homework 2 - Geometric Calculations 100% Satisfaction Guaranteed!
- From Business, Accounting
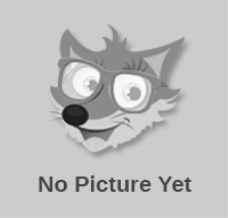
- NUMBER1TUTOR
- Rating : 88
- Grade : A+
- Questions : 0
- Solutions : 0
- Blog : 3
- Earned : $14856.59
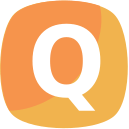
Homework 2 - Geometric Calculations
In this homework assignment, you will be writing an area calculator to understand console
input/output, some basic data types, and basic arithmetic in C++.
This assignment has you
• translating equations into C++ expressions,
• using math constants,
• using math functions,
• precisely formatting output.
Part I: Calculating Areas
1. In Visual C++, create a new project (called, for example, homework2 or HW2 or ...), for this
assignment. Never use spaces in filenames or project names. Follow the same process as
you did in the first assignment to create the project and Add ! New Item to the Source Files
folder, calling it hw2.cpp or somethingsimilar. If you need a refresher, please refer back to
Homework 1. You should copy all of the Hello World code as a starting point. Look at the
last assignment if you need to.
2. We're going to need to include an additional header file for this assignment, one for some
math functions we'll be using. So, include cmath and this #define before the other #include
at the very top of your program file:
#define
_USE_MATH_DEFINES
//
for
C++
#include <cmath>
And use the constant M_PI for π in your equations.
4. You're going to write a small program that prompts the user to enter the radius of a circle,
followed by the length and the width of a rectangle, all in meters. Then, the program
computes the area of the circle, the area of the rectangle, and the total areas of the circle and
the rectangle.
5. We're going to need variables to store values that the user is going to type in. Inside main(),
declare three variables of type double for radius, width, and length.
If you aren't sure how to declare the variables, please refer to the textbook.
6. Remember how we used cout to write text output to the screen? When reading input, you
usually prompt the user with some text using cout. Then, you read the data that the user
responds with using cin. For example, here is what you do for radius:
cout << "Please enter the radius of a circle: ";
cin >> radius;
Notice that there is a space after the colon and no endl at the end of the prompt line. This
means that when you run the program and are asked to enter a number, it will go on the
same line as the prompt. The Enter shown below in the output is from the user hitting
Enter!
Also note the direction of the << and >> for cout and cin!
7. Run the program to make sure you don't have any errors at this point. Adding a few lines
and running it to make sure there are not errors is called incremental development.
8. Now repeat this "prompt and read" process for the width and then for the length of the
rectangle.
9. Next, declare three more variables at the top of the main() of type double to hold the
values your program needs to calculate for circle area, rectangle area, and the total area of
the two combined.
10. The mathematical formulas to calculate the circle area and rectangle area are:
The area of a circle ! π * radius2
The area of a rectangle ! length * width
Your output should look like this: (Please copy and paste the text from these prompts into your
code! You are graded on this on Web-CAT.)
Please enter the radius of a circle: 10
Please enter the width of a rectangle: 20
Please enter the length of a rectangle: 30
The area of the circle is 314.159.
The area of the rectangle is 600.
The combined area is 914.159.
Remember:
copy-paste text
Run your code with several different inputs and hand check the
values to make sure your code is doing the calculations right.
Part II: Adding Circumference and Diagonal Length
Now we will further incrementally add more code to do more!
1. Next, modify the program to calculate the circumference of the circle, the diagonal length of
the rectangle, and the difference of the two lengths.
2. Add three new variable declarations of type double to represent these 3 values.
3. Now, add the code to calculate the circumference of a circle, the diagonal length of
rectangle, and the difference of the two lengths. The mathematical formulas to calculate the
circumference of a circle and the diagonal length of a rectangle are:
circle circumference ! 2 π * radius
rectangle diagonal length ! sqrt ( length2 + width2 )
Sample Output: Your program will be tested with several input sets, including the one
shown here in RED. Your program must exactly match the words and format in this
example. The numbers vary, of course, based on the input numbers.
Please enter the radius of a circle: 10
Please enter the width of a rectangle: 20
Please enter the length of a rectangle: 30
The area of the circle is 314.159.
The area of the rectangle is 600.
The combined area is 914.159.
The circumference of the circle is 62.8319.
The diagonal length of the rectangle is 36.0555.
The difference between the two is 26.7763.
Run your code with several different inputs and hand check the
values to make sure your code is doing the calculations right.
Grading
20% of your grade will be based on proper programming style, formatting, and comments. Although your
initial grade could be 80 out of 80, i.e. 80/80, the remaining 20 points are assignment by a TA. Good style is
described throughout the text. At this point, the most important things are that:
• Use descriptive variable names
• Vertical (proper use of blank line mostly) and horizontal spacing (putting spaces around
operators, indentation, etc.)
o Indent properly
o Skip a line between each small section of your program, so that each section is
separated by a blank line,
• Have a beginning comment that describes what the program is doing (just “Homework 2” is not
good enough). See the main moodle page for what every program header needs to contain.
• Each section (i.e. logicl chunk of code) begins with a comment describing what that section does,
so that the comments alone would provide an outline of the program (It’s NOT good to follow
every line of code with a comment.)
• Continue typing your code on the next line if it is too long. Too long is over 80 characters. In VS,
there is a column indicator at the bottom-right of the screen that tells you which column the cursor
is at.
Note that this is a graded assignment and should be completed on an individual basis!!
Submit Your Work
To submit your work to Web-CAT to be graded. It will only be available on Web-CAT a few days before it is due, so test your code on your own, i.e. make sure the output numbers are correct for a variety of input values.
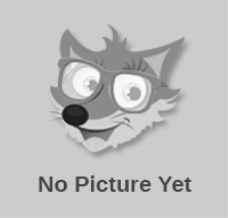
Homework Assignment AA
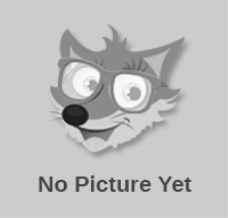
Homework Assignment AA
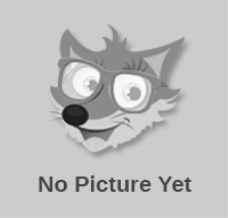
Homework Assignment 3
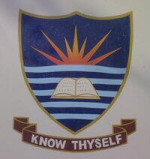
Stat Homework Help
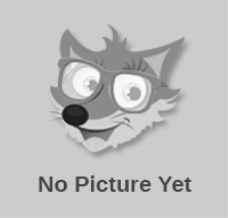
Milestone 2 Assignment
The benefits of buying study notes from CourseMerits
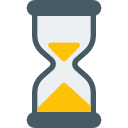
Assurance Of Timely Delivery
We value your patience, and to ensure you always receive your homework help within the promised time, our dedicated team of tutors begins their work as soon as the request arrives.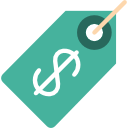
Best Price In The Market
All the services that are available on our page cost only a nominal amount of money. In fact, the prices are lower than the industry standards. You can always expect value for money from us.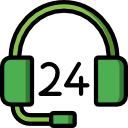